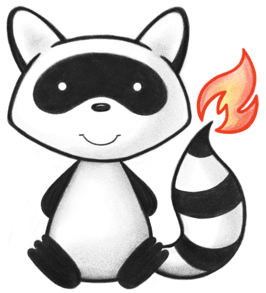
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.api; 021 022import ca.uhn.fhir.util.CoverageIgnore; 023import jakarta.annotation.Nonnull; 024import org.apache.commons.lang3.Validate; 025 026import java.util.HashMap; 027import java.util.Map; 028 029@CoverageIgnore 030public enum RestOperationTypeEnum { 031 BATCH("batch", true, false, false), 032 033 ADD_TAGS("add-tags", false, false, true), 034 035 DELETE_TAGS("delete-tags", false, false, true), 036 037 GET_TAGS("get-tags", false, true, true), 038 039 GET_PAGE("get-page", false, false, false), 040 041 /** 042 * <b> 043 * Use this value with caution, this may 044 * change as the GraphQL interface matures 045 * </b> 046 */ 047 GRAPHQL_REQUEST("graphql-request", false, false, false), 048 049 /** 050 * E.g. $everything, $validate, etc. 051 */ 052 EXTENDED_OPERATION_SERVER("extended-operation-server", false, false, false), 053 054 /** 055 * E.g. $everything, $validate, etc. 056 */ 057 EXTENDED_OPERATION_TYPE("extended-operation-type", false, false, false), 058 059 /** 060 * E.g. $everything, $validate, etc. 061 */ 062 EXTENDED_OPERATION_INSTANCE("extended-operation-instance", false, false, false), 063 064 /** 065 * Code Value: <b>create</b> 066 */ 067 CREATE("create", false, true, false), 068 069 /** 070 * Code Value: <b>delete</b> 071 */ 072 DELETE("delete", false, false, true), 073 074 /** 075 * Code Value: <b>history-instance</b> 076 */ 077 HISTORY_INSTANCE("history-instance", false, false, true), 078 079 /** 080 * Code Value: <b>history-system</b> 081 */ 082 HISTORY_SYSTEM("history-system", true, false, false), 083 084 /** 085 * Code Value: <b>history-type</b> 086 */ 087 HISTORY_TYPE("history-type", false, true, false), 088 089 /** 090 * Code Value: <b>read</b> 091 */ 092 READ("read", false, false, true), 093 094 /** 095 * Code Value: <b>search-system</b> 096 */ 097 SEARCH_SYSTEM("search-system", true, false, false), 098 099 /** 100 * Code Value: <b>search-type</b> 101 */ 102 SEARCH_TYPE("search-type", false, true, false), 103 104 /** 105 * Code Value: <b>transaction</b> 106 */ 107 TRANSACTION("transaction", true, false, false), 108 109 /** 110 * Code Value: <b>update</b> 111 */ 112 UPDATE("update", false, false, true), 113 114 /** 115 * Code Value: <b>validate</b> 116 */ 117 VALIDATE("validate", false, true, true), 118 119 /** 120 * Code Value: <b>vread</b> 121 */ 122 VREAD("vread", false, false, true), 123 124 /** 125 * Load the server's metadata 126 */ 127 METADATA("metadata", false, false, false), 128 129 /** 130 * $meta-add extended operation 131 */ 132 META_ADD("$meta-add", false, false, false), 133 134 /** 135 * $meta-add extended operation 136 */ 137 META("$meta", false, false, false), 138 139 /** 140 * $meta-delete extended operation 141 */ 142 META_DELETE("$meta-delete", false, false, false), 143 144 /** 145 * Patch operation 146 */ 147 PATCH("patch", false, false, true), 148 149 /** 150 * Code Value: <b>update-rewrite-history</b> 151 */ 152 UPDATE_REWRITE_HISTORY("update-rewrite-history", false, false, true), 153 ; 154 155 private static final Map<String, RestOperationTypeEnum> CODE_TO_ENUM = new HashMap<String, RestOperationTypeEnum>(); 156 157 /** 158 * Identifier for this Value Set: http://hl7.org/fhir/vs/type-restful-operation 159 */ 160 public static final String VALUESET_IDENTIFIER = "http://hl7.org/fhir/vs/type-restful-operation"; 161 162 /** 163 * Name for this Value Set: RestfulOperationType 164 */ 165 public static final String VALUESET_NAME = "RestfulOperationType"; 166 167 static { 168 for (RestOperationTypeEnum next : RestOperationTypeEnum.values()) { 169 CODE_TO_ENUM.put(next.getCode(), next); 170 } 171 } 172 173 private final String myCode; 174 private final boolean mySystemLevel; 175 private final boolean myTypeLevel; 176 private final boolean myInstanceLevel; 177 178 /** 179 * Constructor 180 */ 181 RestOperationTypeEnum( 182 @Nonnull String theCode, boolean theSystemLevel, boolean theTypeLevel, boolean theInstanceLevel) { 183 myCode = theCode; 184 mySystemLevel = theSystemLevel; 185 myTypeLevel = theTypeLevel; 186 myInstanceLevel = theInstanceLevel; 187 } 188 189 /** 190 * Returns the enumerated value associated with this code 191 */ 192 public static RestOperationTypeEnum forCode(@Nonnull String theCode) { 193 Validate.notNull(theCode, "theCode must not be null"); 194 return CODE_TO_ENUM.get(theCode); 195 } 196 197 /** 198 * Returns the code associated with this enumerated value 199 */ 200 @Nonnull 201 public String getCode() { 202 return myCode; 203 } 204 205 public boolean isSystemLevel() { 206 return mySystemLevel; 207 } 208 209 public boolean isTypeLevel() { 210 return myTypeLevel; 211 } 212 213 public boolean isInstanceLevel() { 214 return myInstanceLevel; 215 } 216}