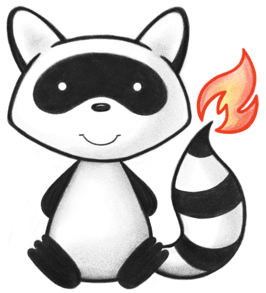
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.api; 021 022import ca.uhn.fhir.model.api.IValueSetEnumBinder; 023 024import java.util.HashMap; 025import java.util.Map; 026 027public enum RestSearchParameterTypeEnum { 028 029 /** 030 * Code Value: <b>number</b> 031 * 032 * Search parameter SHALL be a number (a whole number, or a decimal). 033 */ 034 NUMBER("number", "http://hl7.org/fhir/search-param-type"), 035 036 /** 037 * Code Value: <b>date</b> 038 * 039 * Search parameter is on a date/time. The date format is the standard XML format, though other formats may be supported. 040 */ 041 DATE("date", "http://hl7.org/fhir/search-param-type"), 042 043 /** 044 * Code Value: <b>string</b> 045 * 046 * Search parameter is a simple string, like a name part. Search is case-insensitive and accent-insensitive. May match just the start of a string. String parameters may contain spaces. 047 */ 048 STRING("string", "http://hl7.org/fhir/search-param-type"), 049 050 /** 051 * Code Value: <b>token</b> 052 * 053 * Search parameter on a coded element or identifier. May be used to search through the text, displayname, code and code/codesystem (for codes) and label, system and key (for identifier). Its value is either a string or a pair of namespace and value, separated by a "|", depending on the modifier used. 054 */ 055 TOKEN("token", "http://hl7.org/fhir/search-param-type"), 056 057 /** 058 * Code Value: <b>reference</b> 059 * 060 * A reference to another resource. 061 */ 062 REFERENCE("reference", "http://hl7.org/fhir/search-param-type"), 063 064 /** 065 * Code Value: <b>composite</b> 066 * 067 * A composite search parameter that combines a search on two values together. 068 */ 069 COMPOSITE("composite", "http://hl7.org/fhir/search-param-type"), 070 071 /** 072 * Code Value: <b>quantity</b> 073 * 074 * A search parameter that searches on a quantity. 075 */ 076 QUANTITY("quantity", "http://hl7.org/fhir/search-param-type"), 077 078 /** 079 * Code Value: <b>quantity</b> 080 * 081 * A search parameter that searches on a quantity. 082 */ 083 URI("uri", "http://hl7.org/fhir/search-param-type"), 084 085 /** 086 * _has parameter 087 */ 088 HAS("string", "http://hl7.org/fhir/search-param-type"), 089 090 /** 091 * Code Value: <b>number</b> 092 * 093 * Search parameter SHALL be a number (a whole number, or a decimal). 094 */ 095 SPECIAL("special", "http://hl7.org/fhir/search-param-type"), 096 ; 097 098 /** 099 * Identifier for this Value Set: 100 * http://hl7.org/fhir/vs/search-param-type 101 */ 102 public static final String VALUESET_IDENTIFIER = "http://hl7.org/fhir/vs/search-param-type"; 103 104 /** 105 * Name for this Value Set: 106 * SearchParamType 107 */ 108 public static final String VALUESET_NAME = "SearchParamType"; 109 110 private static Map<String, RestSearchParameterTypeEnum> CODE_TO_ENUM = 111 new HashMap<String, RestSearchParameterTypeEnum>(); 112 private static Map<String, Map<String, RestSearchParameterTypeEnum>> SYSTEM_TO_CODE_TO_ENUM = 113 new HashMap<String, Map<String, RestSearchParameterTypeEnum>>(); 114 115 private final String myCode; 116 private final String mySystem; 117 118 static { 119 for (RestSearchParameterTypeEnum next : RestSearchParameterTypeEnum.values()) { 120 if (next == HAS) { 121 continue; 122 } 123 124 CODE_TO_ENUM.put(next.getCode(), next); 125 126 if (!SYSTEM_TO_CODE_TO_ENUM.containsKey(next.getSystem())) { 127 SYSTEM_TO_CODE_TO_ENUM.put(next.getSystem(), new HashMap<String, RestSearchParameterTypeEnum>()); 128 } 129 SYSTEM_TO_CODE_TO_ENUM.get(next.getSystem()).put(next.getCode(), next); 130 } 131 } 132 133 /** 134 * Returns the code associated with this enumerated value 135 */ 136 public String getCode() { 137 return myCode; 138 } 139 140 /** 141 * Returns the code system associated with this enumerated value 142 */ 143 public String getSystem() { 144 return mySystem; 145 } 146 147 /** 148 * Returns the enumerated value associated with this code 149 */ 150 public static RestSearchParameterTypeEnum forCode(String theCode) { 151 RestSearchParameterTypeEnum retVal = CODE_TO_ENUM.get(theCode); 152 return retVal; 153 } 154 155 /** 156 * Converts codes to their respective enumerated values 157 */ 158 public static final IValueSetEnumBinder<RestSearchParameterTypeEnum> VALUESET_BINDER = 159 new IValueSetEnumBinder<RestSearchParameterTypeEnum>() { 160 private static final long serialVersionUID = 1L; 161 162 @Override 163 public String toCodeString(RestSearchParameterTypeEnum theEnum) { 164 return theEnum.getCode(); 165 } 166 167 @Override 168 public String toSystemString(RestSearchParameterTypeEnum theEnum) { 169 return theEnum.getSystem(); 170 } 171 172 @Override 173 public RestSearchParameterTypeEnum fromCodeString(String theCodeString) { 174 return CODE_TO_ENUM.get(theCodeString); 175 } 176 177 @Override 178 public RestSearchParameterTypeEnum fromCodeString(String theCodeString, String theSystemString) { 179 Map<String, RestSearchParameterTypeEnum> map = SYSTEM_TO_CODE_TO_ENUM.get(theSystemString); 180 if (map == null) { 181 return null; 182 } 183 return map.get(theCodeString); 184 } 185 }; 186 187 /** 188 * Constructor 189 */ 190 RestSearchParameterTypeEnum(String theCode, String theSystem) { 191 myCode = theCode; 192 mySystem = theSystem; 193 } 194}