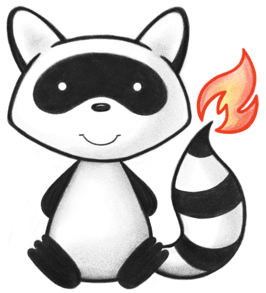
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.api; 021 022import ca.uhn.fhir.i18n.Msg; 023 024import java.io.Serializable; 025 026/** 027 * Represents values for <a href="http://hl7.org/implement/standards/fhir/search.html#sort">sorting</a> resources 028 * returned by a server. 029 */ 030public class SortSpec implements Serializable { 031 032 private static final long serialVersionUID = 2866833099879713467L; 033 034 private SortSpec myChain; 035 private String myParamName; 036 private SortOrderEnum myOrder; 037 038 /** 039 * Constructor 040 */ 041 public SortSpec() { 042 super(); 043 } 044 045 /** 046 * Constructor 047 * 048 * @param theParamName 049 * The search name to sort on. See {@link #setParamName(String)} for more information. 050 */ 051 public SortSpec(String theParamName) { 052 super(); 053 myParamName = theParamName; 054 } 055 056 /** 057 * Constructor 058 * 059 * @param theParamName 060 * The search name to sort on. See {@link #setParamName(String)} for more information. 061 * @param theOrder 062 * The order, or <code>null</code>. See {@link #setOrder(SortOrderEnum)} for more information. 063 */ 064 public SortSpec(String theParamName, SortOrderEnum theOrder) { 065 super(); 066 myParamName = theParamName; 067 myOrder = theOrder; 068 } 069 070 /** 071 * Constructor 072 * 073 * @param theParamName 074 * The search name to sort on. See {@link #setParamName(String)} for more information. 075 * @param theOrder 076 * The order, or <code>null</code>. See {@link #setOrder(SortOrderEnum)} for more information. 077 * @param theChain 078 * The next sorting spec, to be applied only when this spec makes two entries equal. See 079 * {@link #setChain(SortSpec)} for more information. 080 */ 081 public SortSpec(String theParamName, SortOrderEnum theOrder, SortSpec theChain) { 082 super(); 083 myParamName = theParamName; 084 myOrder = theOrder; 085 myChain = theChain; 086 } 087 088 /** 089 * Gets the chained sort specification, or <code>null</code> if none. If multiple sort parameters are chained 090 * (indicating a sub-sort), the second level sort is chained via this property. 091 */ 092 public SortSpec getChain() { 093 return myChain; 094 } 095 096 /** 097 * Returns the actual name of the search param to sort by 098 */ 099 public String getParamName() { 100 return myParamName; 101 } 102 103 /** 104 * Returns the sort order specified by this parameter, or <code>null</code> if none is explicitly provided (which 105 * means {@link SortOrderEnum#ASC} according to the <a 106 * href="http://hl7.org/implement/standards/fhir/search.html#sort">FHIR specification</a>) 107 */ 108 public SortOrderEnum getOrder() { 109 return myOrder; 110 } 111 112 /** 113 * Sets the chained sort specification, or <code>null</code> if none. If multiple sort parameters are chained 114 * (indicating a sub-sort), the second level sort is chained via this property. 115 */ 116 public SortSpec setChain(SortSpec theChain) { 117 if (theChain == this) { 118 throw new IllegalArgumentException(Msg.code(1966) + "Can not chain this to itself"); 119 } 120 myChain = theChain; 121 return this; 122 } 123 124 /** 125 * Sets the actual name of the search param to sort by 126 */ 127 public SortSpec setParamName(String theFieldName) { 128 myParamName = theFieldName; 129 return this; 130 } 131 132 /** 133 * Sets the sort order specified by this parameter, or <code>null</code> if none should be explicitly defined (which 134 * means {@link SortOrderEnum#ASC} according to the <a 135 * href="http://hl7.org/implement/standards/fhir/search.html#sort">FHIR specification</a>) 136 */ 137 public SortSpec setOrder(SortOrderEnum theOrder) { 138 myOrder = theOrder; 139 return this; 140 } 141}