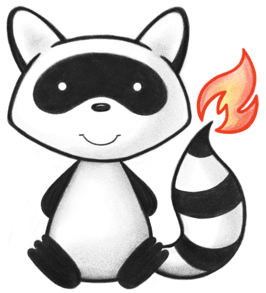
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.api; 021 022import org.hl7.fhir.instance.model.api.IBaseOperationOutcome; 023 024import java.util.ArrayList; 025import java.util.HashMap; 026import java.util.List; 027import java.util.Map; 028 029/** 030 * Response of a http request which can return a String payload 031 */ 032public class StringOutcome implements IHasHeaders { 033 034 private String myPayload; 035 private IBaseOperationOutcome myOperationOutcome; 036 private int myResponseStatus; 037 private Map<String, List<String>> myResponseHeaders; 038 039 public StringOutcome(int theResponseStatus, String thePayload, Map<String, List<String>> theHeaders) { 040 myResponseStatus = theResponseStatus; 041 myPayload = thePayload; 042 myResponseHeaders = theHeaders; 043 } 044 045 public StringOutcome() {} 046 047 /** 048 * Returns the {@link String} payload to return to the client or <code>null</code> if none. 049 * 050 * @return This method <b>may return null</b>, unlike many methods in the API. 051 */ 052 public String getPayload() { 053 return myPayload; 054 } 055 056 /** 057 * Sets the {@link String} payload to return to the client or <code>null</code> if none. 058 */ 059 public void setPayload(String thePayload) { 060 myPayload = thePayload; 061 } 062 063 /** 064 * Returns the {@link IBaseOperationOutcome} resource to return to the client or <code>null</code> if none. 065 * 066 * @return This method <b>will return null</b>, unlike many methods in the API. 067 */ 068 public IBaseOperationOutcome getOperationOutcome() { 069 return myOperationOutcome; 070 } 071 072 /** 073 * Sets the {@link IBaseOperationOutcome} resource to return to the client. Set to <code>null</code> (which is the default) if none. 074 * 075 * @return a reference to <code>this</code> for easy method chaining 076 */ 077 public StringOutcome setOperationOutcome(IBaseOperationOutcome theBaseOperationOutcome) { 078 myOperationOutcome = theBaseOperationOutcome; 079 return this; 080 } 081 082 /** 083 * Gets the headers for the HTTP response 084 */ 085 public Map<String, List<String>> getResponseHeaders() { 086 if (myResponseHeaders == null) { 087 myResponseHeaders = new HashMap<>(); 088 } 089 return myResponseHeaders; 090 } 091 092 /** 093 * Adds a header to the response 094 */ 095 public void addHeader(String theHeaderKey, String theHeaderValue) { 096 myResponseHeaders.getOrDefault(theHeaderKey, new ArrayList<>()).add(theHeaderValue); 097 } 098 099 /** 100 * Gets the HTTP response status 101 */ 102 public int getResponseStatus() { 103 return myResponseStatus; 104 } 105}