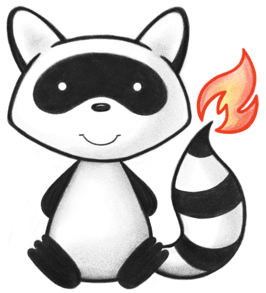
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.api; 021 022import java.util.HashMap; 023import java.util.Map; 024 025/** 026 * Enum representing the values for the <code>_summary</code> search parameter 027 */ 028public enum SummaryEnum { 029 030 /** 031 * Search only: just return a count of the matching resources, without returning the actual matches 032 */ 033 COUNT("count"), 034 035 /** 036 * Return only the 'text' element, and any mandatory elements 037 */ 038 TEXT("text"), 039 040 /** 041 * Remove the text element 042 */ 043 DATA("data"), 044 045 /** 046 * Return only those elements marked as 'summary' in the base definition of the resource(s) 047 */ 048 TRUE("true"), 049 050 /** 051 * Return all parts of the resource(s) 052 */ 053 FALSE("false"); 054 055 private String myCode; 056 private static Map<String, SummaryEnum> ourCodeToSummary = null; 057 058 SummaryEnum(String theCode) { 059 myCode = theCode; 060 } 061 062 public String getCode() { 063 return myCode; 064 } 065 066 public static SummaryEnum fromCode(String theCode) { 067 Map<String, SummaryEnum> c2s = ourCodeToSummary; 068 if (c2s == null) { 069 c2s = new HashMap<>(); 070 for (SummaryEnum next : values()) { 071 c2s.put(next.getCode(), next); 072 } 073 ourCodeToSummary = c2s; 074 } 075 return c2s.get(theCode); 076 } 077}