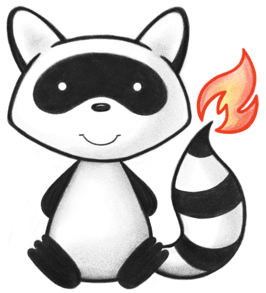
001/*- 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.client.api; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.interceptor.api.Pointcut; 024import org.hl7.fhir.instance.model.api.IBaseResource; 025 026import java.util.Objects; 027import java.util.StringJoiner; 028 029/** 030 * Used to pass context to {@link Pointcut#CLIENT_RESPONSE}, including a mutable {@link IHttpResponse} 031 */ 032public class ClientResponseContext { 033 private final IHttpRequest myHttpRequest; 034 private IHttpResponse myHttpResponse; 035 private final IRestfulClient myRestfulClient; 036 private final FhirContext myFhirContext; 037 private final Class<? extends IBaseResource> myReturnType; 038 039 public ClientResponseContext( 040 IHttpRequest myHttpRequest, 041 IHttpResponse theHttpResponse, 042 IRestfulClient myRestfulClient, 043 FhirContext theFhirContext, 044 Class<? extends IBaseResource> theReturnType) { 045 this.myHttpRequest = myHttpRequest; 046 this.myHttpResponse = theHttpResponse; 047 this.myRestfulClient = myRestfulClient; 048 this.myFhirContext = theFhirContext; 049 this.myReturnType = theReturnType; 050 } 051 052 public IHttpRequest getHttpRequest() { 053 return myHttpRequest; 054 } 055 056 public IHttpResponse getHttpResponse() { 057 return myHttpResponse; 058 } 059 060 public IRestfulClient getRestfulClient() { 061 return myRestfulClient; 062 } 063 064 public FhirContext getFhirContext() { 065 return myFhirContext; 066 } 067 068 public Class<? extends IBaseResource> getReturnType() { 069 return myReturnType; 070 } 071 072 public void setHttpResponse(IHttpResponse theHttpResponse) { 073 this.myHttpResponse = theHttpResponse; 074 } 075 076 @Override 077 public boolean equals(Object o) { 078 if (this == o) return true; 079 if (o == null || getClass() != o.getClass()) return false; 080 ClientResponseContext that = (ClientResponseContext) o; 081 return Objects.equals(myHttpRequest, that.myHttpRequest) 082 && Objects.equals(myHttpResponse, that.myHttpResponse) 083 && Objects.equals(myRestfulClient, that.myRestfulClient) 084 && Objects.equals(myFhirContext, that.myFhirContext) 085 && Objects.equals(myReturnType, that.myReturnType); 086 } 087 088 @Override 089 public int hashCode() { 090 return Objects.hash(myHttpRequest, myHttpResponse, myRestfulClient, myFhirContext, myReturnType); 091 } 092 093 @Override 094 public String toString() { 095 return new StringJoiner(", ", ClientResponseContext.class.getSimpleName() + "[", "]") 096 .add("myHttpRequest=" + myHttpRequest) 097 .add("myHttpResponse=" + myHttpResponse) 098 .add("myRestfulClient=" + myRestfulClient) 099 .add("myFhirContext=" + myFhirContext) 100 .add("myReturnType=" + myReturnType) 101 .toString(); 102 } 103}