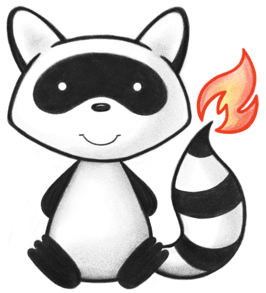
001/*- 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.client.api; 021 022import ca.uhn.fhir.model.primitive.IdDt; 023import ca.uhn.fhir.model.primitive.UriDt; 024import ca.uhn.fhir.rest.api.MethodOutcome; 025import ca.uhn.fhir.rest.client.exceptions.FhirClientConnectionException; 026import ca.uhn.fhir.rest.client.exceptions.FhirClientInappropriateForServerException; 027import ca.uhn.fhir.rest.gclient.ICreate; 028import ca.uhn.fhir.rest.gclient.IDelete; 029import ca.uhn.fhir.rest.gclient.IFetchConformanceUntyped; 030import ca.uhn.fhir.rest.gclient.IGetPage; 031import ca.uhn.fhir.rest.gclient.IHistory; 032import ca.uhn.fhir.rest.gclient.IMeta; 033import ca.uhn.fhir.rest.gclient.IOperation; 034import ca.uhn.fhir.rest.gclient.IPatch; 035import ca.uhn.fhir.rest.gclient.IRead; 036import ca.uhn.fhir.rest.gclient.ITransaction; 037import ca.uhn.fhir.rest.gclient.IUntypedQuery; 038import ca.uhn.fhir.rest.gclient.IUpdate; 039import ca.uhn.fhir.rest.gclient.IValidate; 040import org.hl7.fhir.instance.model.api.IBaseBundle; 041import org.hl7.fhir.instance.model.api.IBaseResource; 042 043public interface IGenericClient extends IRestfulClient { 044 045 /** 046 * Fetch the capability statement for the server 047 */ 048 IFetchConformanceUntyped capabilities(); 049 050 /** 051 * Fluent method for the "create" operation, which creates a new resource instance on the server 052 */ 053 ICreate create(); 054 055 /** 056 * Fluent method for the "delete" operation, which performs a logical delete on a server resource 057 */ 058 IDelete delete(); 059 060 /** 061 * Retrieves the server's conformance statement 062 * 063 * @deprecated As of HAPI 3.0.0 this method has been deprecated, as the operation is now called "capabilities". Use {@link #capabilities()} instead 064 */ 065 @Deprecated(since = "3.0.0", forRemoval = true) 066 IFetchConformanceUntyped fetchConformance(); 067 068 /** 069 * Force the client to fetch the server's conformance statement and validate that it is appropriate for this client. 070 * 071 * @throws FhirClientConnectionException 072 * if the conformance statement cannot be read, or if the client 073 * @throws FhirClientInappropriateForServerException 074 * If the conformance statement indicates that the server is inappropriate for this client (e.g. it implements the wrong version of FHIR) 075 */ 076 void forceConformanceCheck() throws FhirClientConnectionException; 077 078 /** 079 * Implementation of the "history" method 080 */ 081 IHistory history(); 082 083 /** 084 * Loads the previous/next bundle of resources from a paged set, using the link specified in the "link type=next" tag within the atom bundle. 085 */ 086 IGetPage loadPage(); 087 088 /** 089 * Fluent method for the "meta" operations, which can be used to get, add and remove tags and other 090 * Meta elements from a resource or across the server. 091 * 092 * @since 1.1 093 */ 094 IMeta meta(); 095 096 /** 097 * Implementation of the FHIR "extended operations" action 098 */ 099 IOperation operation(); 100 101 /** 102 * Fluent method for the "patch" operation, which performs a logical patch on a server resource 103 */ 104 IPatch patch(); 105 106 /** 107 * Fluent method for "read" and "vread" methods. 108 */ 109 IRead read(); 110 111 /** 112 * Implementation of the "instance read" method. 113 * 114 * @param theType 115 * The type of resource to load 116 * @param theId 117 * The ID to load 118 * @return The resource 119 * 120 * @deprecated Use {@link #read() read() fluent method} instead (deprecated in HAPI FHIR 3.0.0) 121 */ 122 @Deprecated 123 <T extends IBaseResource> T read(Class<T> theType, String theId); 124 125 /** 126 * Perform the "read" operation (retrieve the latest version of a resource instance by ID) using an absolute URL. 127 * 128 * @param theType 129 * The resource type that is being retrieved 130 * @param theUrl 131 * The absolute URL, e.g. "http://example.com/fhir/Patient/123" 132 * @return The returned resource from the server 133 * @deprecated Use {@link #read() read() fluent method} instead (deprecated in HAPI FHIR 3.0.0) 134 */ 135 @Deprecated 136 <T extends IBaseResource> T read(Class<T> theType, UriDt theUrl); 137 138 /** 139 * Perform the "read" operation (retrieve the latest version of a resource instance by ID) using an absolute URL. 140 * 141 * @param theUrl 142 * The absolute URL, e.g. "http://example.com/fhir/Patient/123" 143 * @return The returned resource from the server 144 * @deprecated Use {@link #read() read() fluent method} instead (deprecated in HAPI FHIR 3.0.0) 145 */ 146 @Deprecated 147 IBaseResource read(UriDt theUrl); 148 149 /** 150 * Register a new interceptor for this client. An interceptor can be used to add additional logging, or add security headers, or pre-process responses, etc. 151 */ 152 @Override 153 void registerInterceptor(Object theInterceptor); 154 155 /** 156 * Search for resources matching a given set of criteria. Searching is a very powerful 157 * feature in FHIR with many features for specifying exactly what should be seaerched for 158 * and how it should be returned. See the <a href="http://www.hl7.org/fhir/search.html">specification on search</a> 159 * for more information. 160 */ 161 <T extends IBaseBundle> IUntypedQuery<T> search(); 162 163 /** 164 * If set to <code>true</code>, the client will log all requests and all responses. This is probably not a good production setting since it will result in a lot of extra logging, but it can be 165 * useful for troubleshooting. 166 * 167 * @param theLogRequestAndResponse 168 * Should requests and responses be logged 169 * @deprecated Use LoggingInterceptor as a client interceptor registered to your 170 * client instead, as this provides much more fine-grained control over what is logged. This 171 * method will be removed at some point (deprecated in HAPI 1.6 - 2016-06-16) 172 */ 173 @Deprecated 174 void setLogRequestAndResponse(boolean theLogRequestAndResponse); 175 176 /** 177 * Send a transaction (collection of resources) to the server to be executed as a single unit 178 */ 179 ITransaction transaction(); 180 181 /** 182 * Remove an intercaptor that was previously registered using {@link IRestfulClient#registerInterceptor(Object)} 183 */ 184 @Override 185 void unregisterInterceptor(Object theInterceptor); 186 187 /** 188 * Fluent method for the "update" operation, which updates a resource instance on the server 189 */ 190 IUpdate update(); 191 192 /** 193 * Implementation of the "instance update" method. 194 * 195 * @param theId 196 * The ID to update 197 * @param theResource 198 * The new resource body 199 * @return An outcome containing the results and possibly the new version ID 200 * @deprecated Use {@link #update() update() fluent method} instead (deprecated in HAPI FHIR 3.0.0) 201 */ 202 @Deprecated 203 MethodOutcome update(IdDt theId, IBaseResource theResource); 204 205 /** 206 * Implementation of the "instance update" method. 207 * 208 * @param theId 209 * The ID to update 210 * @param theResource 211 * The new resource body 212 * @return An outcome containing the results and possibly the new version ID 213 * @deprecated Use {@link #update() update() fluent method} instead (deprecated in HAPI FHIR 3.0.0) 214 */ 215 @Deprecated 216 MethodOutcome update(String theId, IBaseResource theResource); 217 218 /** 219 * Validate a resource 220 */ 221 IValidate validate(); 222 223 /** 224 * Implementation of the "type validate" method. 225 * 226 * @param theResource 227 * The resource to validate 228 * @return An outcome containing any validation issues 229 * @deprecated Use {@link #validate() validate() fluent method} instead (deprecated in HAPI FHIR 3.0.0) 230 */ 231 @Deprecated 232 MethodOutcome validate(IBaseResource theResource); 233 234 /** 235 * Implementation of the "instance vread" method. Note that this method expects <code>theId</code> to contain a resource ID as well as a version ID, and will fail if it does not. 236 * <p> 237 * Note that if an absolute resource ID is passed in (i.e. a URL containing a protocol and host as well as the resource type and ID) the server base for the client will be ignored, and the URL 238 * passed in will be queried. 239 * </p> 240 * 241 * @param theType 242 * The type of resource to load 243 * @param theId 244 * The ID to load, including the resource ID and the resource version ID. Valid values include "Patient/123/_history/222", or "http://example.com/fhir/Patient/123/_history/222" 245 * @return The resource 246 * @deprecated Use {@link #read() read() fluent method} instead (deprecated in HAPI FHIR 3.0.0) 247 */ 248 @Deprecated 249 <T extends IBaseResource> T vread(Class<T> theType, IdDt theId); 250 251 /** 252 * Implementation of the "instance vread" method. 253 * 254 * @param theType 255 * The type of resource to load 256 * @param theId 257 * The ID to load 258 * @param theVersionId 259 * The version ID 260 * @return The resource 261 * @deprecated Use {@link #read() read() fluent method} instead (deprecated in HAPI FHIR 3.0.0) 262 */ 263 @Deprecated 264 <T extends IBaseResource> T vread(Class<T> theType, String theId, String theVersionId); 265}