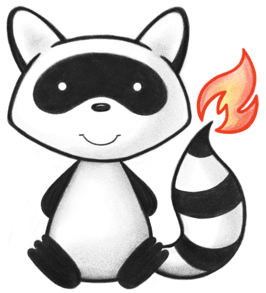
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.client.api; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.rest.api.EncodingEnum; 024import org.hl7.fhir.instance.model.api.IBaseBinary; 025 026import java.util.List; 027import java.util.Map; 028 029/** 030 * A HTTP Client interface. 031 */ 032public interface IHttpClient { 033 034 /** 035 * Create a byte request 036 * @param theContext TODO 037 * @param theContents the contents 038 * @param theContentType the contentType 039 * @param theEncoding the encoding 040 * @return the http request to be executed 041 */ 042 IHttpRequest createByteRequest( 043 FhirContext theContext, String theContents, String theContentType, EncodingEnum theEncoding); 044 045 /** 046 * Create a parameter request 047 * @param theContext TODO 048 * @param theParams the parameters 049 * @param theEncoding the encoding 050 * @return the http request to be executed 051 */ 052 IHttpRequest createParamRequest( 053 FhirContext theContext, Map<String, List<String>> theParams, EncodingEnum theEncoding); 054 055 /** 056 * Create a binary request 057 * @param theContext TODO 058 * @param theBinary the binary 059 * @return the http request to be executed 060 */ 061 IHttpRequest createBinaryRequest(FhirContext theContext, IBaseBinary theBinary); 062 063 /** 064 * Create a normal http get request 065 * @param theContext TODO 066 * @param theEncoding the request encoding 067 * @return the http request to be executed 068 */ 069 IHttpRequest createGetRequest(FhirContext theContext, EncodingEnum theEncoding); 070}