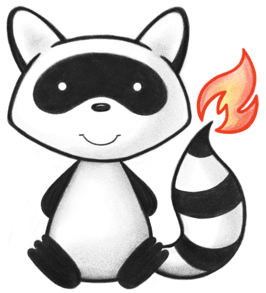
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.client.api; 021 022import java.io.IOException; 023import java.util.List; 024import java.util.Map; 025 026/** 027 * Http Request. Allows addition of headers and execution of the request. 028 */ 029public interface IHttpRequest { 030 031 /** 032 * Add a header to the request 033 * 034 * @param theName the header name 035 * @param theValue the header value 036 */ 037 void addHeader(String theName, String theValue); 038 039 /** 040 * Execute the request 041 * 042 * @return the response 043 */ 044 IHttpResponse execute() throws IOException; 045 046 /** 047 * @return all request headers in lower case. Note that this method 048 * returns an <b>immutable</b> Map 049 */ 050 Map<String, List<String>> getAllHeaders(); 051 052 /** 053 * Return the request body as a string. 054 * If this is not supported by the underlying technology, null is returned 055 * 056 * @return a string representation of the request or null if not supported or empty. 057 */ 058 String getRequestBodyFromStream() throws IOException; 059 060 /** 061 * Return the request URI, or null 062 * 063 * @see #getUri() 064 */ 065 String getUri(); 066 067 /** 068 * Modify the request URI, or null 069 * 070 * @see #setUrlSource(UrlSourceEnum) 071 */ 072 void setUri(String theUrl); 073 074 /** 075 * Return the HTTP verb (e.g. "GET") 076 */ 077 String getHttpVerbName(); 078 079 /** 080 * Remove any headers matching the given name 081 * 082 * @param theHeaderName The header name, e.g. "Accept" (must not be null or blank) 083 */ 084 void removeHeaders(String theHeaderName); 085 086 /** 087 * Where was the URL from? 088 * 089 * @since 5.0.0 090 */ 091 UrlSourceEnum getUrlSource(); 092 093 /** 094 * Where was the URL from? 095 * 096 * @since 5.0.0 097 */ 098 void setUrlSource(UrlSourceEnum theUrlSource); 099}