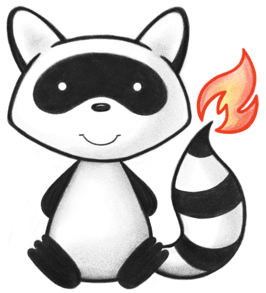
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.client.api; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.interceptor.api.IInterceptorService; 024import ca.uhn.fhir.rest.api.EncodingEnum; 025import ca.uhn.fhir.rest.api.RequestFormatParamStyleEnum; 026import ca.uhn.fhir.rest.api.SummaryEnum; 027import jakarta.annotation.Nonnull; 028import org.hl7.fhir.instance.model.api.IBaseResource; 029 030public interface IRestfulClient { 031 032 /** 033 * Sets the interfceptor service used by this client 034 * 035 * @since 3.8.0 036 */ 037 IInterceptorService getInterceptorService(); 038 039 /** 040 * Sets the interfceptor service used by this client 041 * 042 * @since 3.8.0 043 */ 044 void setInterceptorService(@Nonnull IInterceptorService theInterceptorService); 045 046 /** 047 * Retrieve the contents at the given URL and parse them as a resource. This 048 * method could be used as a low level implementation of a read/vread/search 049 * operation. 050 * 051 * @param theResourceType The resource type to parse 052 * @param theUrl The URL to load 053 * @return The parsed resource 054 */ 055 <T extends IBaseResource> T fetchResourceFromUrl(Class<T> theResourceType, String theUrl); 056 057 /** 058 * Returns the encoding that will be used on requests. Default is <code>null</code>, which means the client will not 059 * explicitly request an encoding. (This is standard behaviour according to the FHIR specification) 060 */ 061 EncodingEnum getEncoding(); 062 063 /** 064 * Specifies that the client should use the given encoding to do its 065 * queries. This means that the client will append the "_format" param 066 * to GET methods (read/search/etc), and will add an appropriate header for 067 * write methods. 068 * 069 * @param theEncoding The encoding to use in the request, or <code>null</code> not specify 070 * an encoding (which generally implies the use of XML). The default is <code>null</code>. 071 */ 072 void setEncoding(EncodingEnum theEncoding); 073 074 /** 075 * Returns the FHIR context associated with this client 076 */ 077 FhirContext getFhirContext(); 078 079 /** 080 * Do not call this method in client code. It is a part of the internal HAPI API and 081 * is subject to change! 082 */ 083 IHttpClient getHttpClient(); 084 085 /** 086 * Base URL for the server, with no trailing "/" 087 */ 088 String getServerBase(); 089 090 /** 091 * Register a new interceptor for this client. An interceptor can be used to add additional 092 * logging, or add security headers, or pre-process responses, etc. 093 * <p> 094 * This is a convenience method for performing the following call: 095 * <code>getInterceptorService().registerInterceptor(theInterceptor)</code> 096 * </p> 097 */ 098 void registerInterceptor(Object theInterceptor); 099 100 /** 101 * Specifies that the client should request that the server respond with "pretty printing" 102 * enabled. Note that this is a non-standard parameter, not all servers will 103 * support it. 104 * 105 * @param thePrettyPrint The pretty print flag to use in the request (default is <code>false</code>) 106 */ 107 void setPrettyPrint(Boolean thePrettyPrint); 108 109 /** 110 * If not set to <code>null</code>, specifies a value for the <code>_summary</code> parameter 111 * to be applied globally on this client. 112 */ 113 void setSummary(SummaryEnum theSummary); 114 115 /** 116 * Remove an interceptor that was previously registered using {@link IRestfulClient#registerInterceptor(Object)}. 117 * <p> 118 * This is a convenience method for performing the following call: 119 * <code>getInterceptorService().unregisterInterceptor(theInterceptor)</code> 120 * </p> 121 */ 122 void unregisterInterceptor(Object theInterceptor); 123 124 /** 125 * Configures what style of _format parameter should be used in requests 126 */ 127 void setFormatParamStyle(RequestFormatParamStyleEnum theRequestFormatParamStyle); 128}