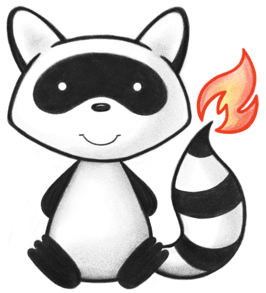
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.client.api; 021 022import ca.uhn.fhir.context.ConfigurationException; 023import ca.uhn.fhir.rest.api.RequestTypeEnum; 024 025import java.util.List; 026import java.util.Map; 027 028public interface IRestfulClientFactory { 029 030 /** 031 * Default value for {@link #getConnectTimeout()} 032 */ 033 public static final int DEFAULT_CONNECT_TIMEOUT = 10000; 034 035 /** 036 * Default value for {@link #getConnectionRequestTimeout()} 037 */ 038 public static final int DEFAULT_CONNECTION_REQUEST_TIMEOUT = 10000; 039 040 /** 041 * Default value for {@link #getConnectionTimeToLive()} 042 */ 043 public static final int DEFAULT_CONNECTION_TTL = 5000; 044 045 /** 046 * Default value for {@link #getServerValidationModeEnum()} 047 */ 048 public static final ServerValidationModeEnum DEFAULT_SERVER_VALIDATION_MODE = ServerValidationModeEnum.ONCE; 049 050 /** 051 * Default value for {@link #getSocketTimeout()} 052 */ 053 public static final int DEFAULT_SOCKET_TIMEOUT = 10000; 054 055 /** 056 * Default value for {@link #getPoolMaxTotal() ()} 057 */ 058 public static final int DEFAULT_POOL_MAX = 20; 059 060 /** 061 * Default value for {@link #getPoolMaxPerRoute() } 062 */ 063 public static final int DEFAULT_POOL_MAX_PER_ROUTE = DEFAULT_POOL_MAX; 064 065 /** 066 * Gets the connection request timeout, in milliseconds. This is the amount of time that the HTTPClient connection 067 * pool may wait for an available connection before failing. This setting typically does not need to be adjusted. 068 * <p> 069 * The default value for this setting is defined by {@link #DEFAULT_CONNECTION_REQUEST_TIMEOUT} 070 * </p> 071 */ 072 int getConnectionRequestTimeout(); 073 074 /** 075 * Gets the connect timeout, in milliseconds. This is the amount of time that the initial connection attempt network 076 * operation may block without failing. 077 * <p> 078 * The default value for this setting is defined by {@link #DEFAULT_CONNECT_TIMEOUT} 079 * </p> 080 */ 081 int getConnectTimeout(); 082 083 /** 084 * Gets the connection time to live, in milliseconds. This is the amount of time to keep connections alive for reuse. 085 * <p> 086 * The default value for this setting is defined by {@link #DEFAULT_CONNECTION_TTL} 087 * </p> 088 */ 089 default int getConnectionTimeToLive() { 090 return DEFAULT_CONNECTION_TTL; 091 } 092 093 /** 094 * Returns the HTTP client instance. This method will not return null. 095 * @param theUrl 096 * The complete FHIR url to which the http request will be sent 097 * @param theIfNoneExistParams 098 * The params for header "If-None-Exist" as a hashmap 099 * @param theIfNoneExistString 100 * The param for header "If-None-Exist" as a string 101 * @param theRequestType 102 * the type of HTTP request (GET, DELETE, ..) 103 * @param theHeaders 104 * the headers to be sent together with the http request 105 * @return the HTTP client instance 106 */ 107 IHttpClient getHttpClient( 108 StringBuilder theUrl, 109 Map<String, List<String>> theIfNoneExistParams, 110 String theIfNoneExistString, 111 RequestTypeEnum theRequestType, 112 List<Header> theHeaders); 113 114 /** 115 * @deprecated Use {@link #getServerValidationMode()} instead (this method is a synonym for that method, but this method is poorly named and will be removed at some point) 116 */ 117 @Deprecated 118 ServerValidationModeEnum getServerValidationModeEnum(); 119 120 /** 121 * Gets the server validation mode for any clients created from this factory. Server 122 * validation involves the client requesting the server's conformance statement 123 * to determine whether the server is appropriate for the given client. 124 * <p> 125 * The default value for this setting is defined by {@link #DEFAULT_SERVER_VALIDATION_MODE} 126 * </p> 127 * 128 * @since 1.0 129 */ 130 ServerValidationModeEnum getServerValidationMode(); 131 132 /** 133 * Gets the socket timeout, in milliseconds. This is the SO_TIMEOUT time, which is the amount of time that a 134 * read/write network operation may block without failing. 135 * <p> 136 * The default value for this setting is defined by {@link #DEFAULT_SOCKET_TIMEOUT} 137 * </p> 138 */ 139 int getSocketTimeout(); 140 141 /** 142 * Gets the maximum number of connections allowed in the pool. 143 * <p> 144 * The default value for this setting is defined by {@link #DEFAULT_POOL_MAX} 145 * </p> 146 */ 147 int getPoolMaxTotal(); 148 149 /** 150 * Gets the maximum number of connections per route allowed in the pool. 151 * <p> 152 * The default value for this setting is defined by {@link #DEFAULT_POOL_MAX_PER_ROUTE} 153 * </p> 154 */ 155 int getPoolMaxPerRoute(); 156 157 /** 158 * Instantiates a new client instance 159 * 160 * @param theClientType 161 * The client type, which is an interface type to be instantiated 162 * @param theServerBase 163 * The URL of the base for the restful FHIR server to connect to 164 * @return A newly created client 165 * @throws ConfigurationException 166 * If the interface type is not an interface 167 */ 168 <T extends IRestfulClient> T newClient(Class<T> theClientType, String theServerBase); 169 170 /** 171 * Instantiates a new generic client instance 172 * 173 * @param theServerBase 174 * The URL of the base for the restful FHIR server to connect to 175 * @return A newly created client 176 */ 177 IGenericClient newGenericClient(String theServerBase); 178 179 /** 180 * Sets the connection request timeout, in milliseconds. This is the amount of time that the HTTPClient connection 181 * pool may wait for an available connection before failing. This setting typically does not need to be adjusted. 182 * <p> 183 * The default value for this setting is defined by {@link #DEFAULT_CONNECTION_REQUEST_TIMEOUT} 184 * </p> 185 */ 186 void setConnectionRequestTimeout(int theConnectionRequestTimeout); 187 188 /** 189 * Sets the connect timeout, in milliseconds. This is the amount of time that the initial connection attempt network 190 * operation may block without failing. 191 * <p> 192 * The default value for this setting is defined by {@link #DEFAULT_CONNECT_TIMEOUT} 193 * </p> 194 */ 195 void setConnectTimeout(int theConnectTimeout); 196 197 /** 198 * Sets the connection time to live, in milliseconds. This is the amount of time to keep connections alive for reuse. 199 * <p> 200 * The default value for this setting is defined by {@link #DEFAULT_CONNECTION_TTL} 201 * </p> 202 */ 203 default void setConnectionTimeToLive(int theConnectionTimeToLive) {} 204 205 /** 206 * Sets the Apache HTTP client instance to be used by any new restful clients created by this factory. If set to 207 * <code>null</code>, a new HTTP client with default settings will be created. 208 * 209 * @param theHttpClient 210 * An HTTP client instance to use, or <code>null</code> 211 */ 212 <T> void setHttpClient(T theHttpClient); 213 214 /** 215 * Sets the HTTP proxy to use for outgoing connections 216 * 217 * @param theHost 218 * The host (or null to disable proxying, as is the default) 219 * @param thePort 220 * The port (or null to disable proxying, as is the default) 221 */ 222 void setProxy(String theHost, Integer thePort); 223 224 /** 225 * Sets the credentials to use to authenticate with the HTTP proxy, 226 * if one is defined. Set to null to use no authentication with the proxy. 227 * @param theUsername The username 228 * @param thePassword The password 229 */ 230 void setProxyCredentials(String theUsername, String thePassword); 231 232 /** 233 * @deprecated Use {@link #setServerValidationMode(ServerValidationModeEnum)} instead. This method was incorrectly named. 234 */ 235 @Deprecated 236 void setServerValidationModeEnum(ServerValidationModeEnum theServerValidationMode); 237 238 /** 239 * Sets the server validation mode for any clients created from this factory. Server 240 * validation involves the client requesting the server's conformance statement 241 * to determine whether the server is appropriate for the given client. 242 * <p> 243 * This check is primarily to validate that the server supports an appropriate 244 * version of FHIR 245 * </p> 246 * <p> 247 * The default value for this setting is defined by {@link #DEFAULT_SERVER_VALIDATION_MODE} 248 * </p> 249 * 250 * @since 1.0 251 */ 252 void setServerValidationMode(ServerValidationModeEnum theServerValidationMode); 253 254 /** 255 * Sets the socket timeout, in milliseconds. This is the SO_TIMEOUT time, which is the amount of time that a 256 * read/write network operation may block without failing. 257 * <p> 258 * The default value for this setting is defined by {@link #DEFAULT_SOCKET_TIMEOUT} 259 * </p> 260 */ 261 void setSocketTimeout(int theSocketTimeout); 262 263 /** 264 * Sets the maximum number of connections allowed in the pool. 265 * <p> 266 * The default value for this setting is defined by {@link #DEFAULT_POOL_MAX} 267 * </p> 268 */ 269 void setPoolMaxTotal(int thePoolMaxTotal); 270 271 /** 272 * Sets the maximum number of connections per route allowed in the pool. 273 * <p> 274 * The default value for this setting is defined by {@link #DEFAULT_POOL_MAX_PER_ROUTE} 275 * </p> 276 */ 277 void setPoolMaxPerRoute(int thePoolMaxPerRoute); 278 279 void validateServerBase(String theServerBase, IHttpClient theHttpClient, IRestfulClient theClient); 280 281 /** 282 * This method is internal to HAPI - It may change in future versions, use with caution. 283 */ 284 void validateServerBaseIfConfiguredToDoSo( 285 String theServerBase, IHttpClient theHttpClient, IRestfulClient theClient); 286}