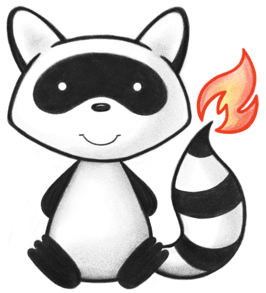
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.client.exceptions; 021 022import ca.uhn.fhir.rest.server.exceptions.BaseServerResponseException; 023import ca.uhn.fhir.util.CoverageIgnore; 024import com.google.common.base.Charsets; 025import org.apache.commons.io.IOUtils; 026 027import java.io.IOException; 028import java.io.InputStream; 029import java.io.InputStreamReader; 030import java.io.Reader; 031 032import static org.apache.commons.lang3.StringUtils.isBlank; 033 034@CoverageIgnore 035public class NonFhirResponseException extends BaseServerResponseException { 036 037 private static final long serialVersionUID = 1L; 038 039 /** 040 * Constructor 041 * 042 * @param theMessage The message 043 * @param theStatusCode The HTTP status code 044 */ 045 NonFhirResponseException(int theStatusCode, String theMessage) { 046 super(theStatusCode, theMessage); 047 } 048 049 public static NonFhirResponseException newInstance( 050 int theStatusCode, String theContentType, InputStream theInputStream) { 051 return newInstance(theStatusCode, theContentType, new InputStreamReader(theInputStream, Charsets.UTF_8)); 052 } 053 054 public static NonFhirResponseException newInstance(int theStatusCode, String theContentType, Reader theReader) { 055 String responseBody = ""; 056 try { 057 responseBody = IOUtils.toString(theReader); 058 } catch (IOException e) { 059 // ignore 060 } finally { 061 try { 062 theReader.close(); 063 } catch (IOException theE) { 064 // ignore 065 } 066 } 067 068 NonFhirResponseException retVal; 069 if (isBlank(theContentType)) { 070 retVal = new NonFhirResponseException(theStatusCode, "Response contains no Content-Type"); 071 } else if (theContentType.contains("text")) { 072 retVal = new NonFhirResponseException( 073 theStatusCode, 074 "Response contains non FHIR Content-Type '" + theContentType + "' : " + responseBody); 075 } else { 076 retVal = new NonFhirResponseException( 077 theStatusCode, "Response contains non FHIR Content-Type '" + theContentType + "'"); 078 } 079 080 retVal.setResponseBody(responseBody); 081 return retVal; 082 } 083}