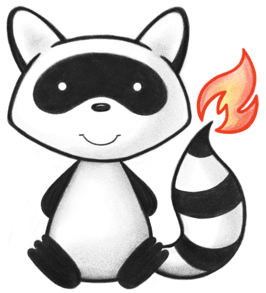
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.gclient; 021 022import ca.uhn.fhir.rest.api.CacheControlDirective; 023import ca.uhn.fhir.rest.api.EncodingEnum; 024import ca.uhn.fhir.rest.api.RequestFormatParamStyleEnum; 025import ca.uhn.fhir.rest.api.SummaryEnum; 026import org.hl7.fhir.instance.model.api.IBaseResource; 027 028import java.util.List; 029 030public interface IClientExecutable<T extends IClientExecutable<?, Y>, Y> { 031 032 /** 033 * If set to true, the client will log the request and response to the SLF4J logger. This can be useful for 034 * debugging, but is generally not desirable in a production situation. 035 * 036 * @deprecated Use the client logging interceptor to log requests and responses instead. See <a href="https://hapifhir.io/hapi-fhir/docs/interceptors/built_in_client_interceptors.html">here</a> for more information. 037 */ 038 @Deprecated 039 T andLogRequestAndResponse(boolean theLogRequestAndResponse); 040 041 /** 042 * Sets the <code>Cache-Control</code> header value, which advises the server (or any cache in front of it) 043 * how to behave in terms of cached requests 044 */ 045 T cacheControl(CacheControlDirective theCacheControlDirective); 046 047 /** 048 * Request that the server return subsetted resources, containing only the elements specified in the given parameters. 049 * For example: <code>subsetElements("name", "identifier")</code> requests that the server only return 050 * the "name" and "identifier" fields in the returned resource, and omit any others. 051 */ 052 T elementsSubset(String... theElements); 053 054 /** 055 * Request that the server respond with JSON via the Accept header and possibly also the 056 * <code>_format</code> parameter if {@link ca.uhn.fhir.rest.client.api.IRestfulClient#setFormatParamStyle(RequestFormatParamStyleEnum) configured to do so}. 057 * <p> 058 * This method will have no effect if {@link #accept(String) a custom Accept header} is specified. 059 * </p> 060 * 061 * @see #accept(String) 062 */ 063 T encoded(EncodingEnum theEncoding); 064 065 /** 066 * Request that the server respond with JSON via the Accept header and possibly also the 067 * <code>_format</code> parameter if {@link ca.uhn.fhir.rest.client.api.IRestfulClient#setFormatParamStyle(RequestFormatParamStyleEnum) configured to do so}. 068 * <p> 069 * This method will have no effect if {@link #accept(String) a custom Accept header} is specified. 070 * </p> 071 * 072 * @see #accept(String) 073 * @see #encoded(EncodingEnum) 074 */ 075 T encodedJson(); 076 077 /** 078 * Request that the server respond with JSON via the Accept header and possibly also the 079 * <code>_format</code> parameter if {@link ca.uhn.fhir.rest.client.api.IRestfulClient#setFormatParamStyle(RequestFormatParamStyleEnum) configured to do so}. 080 * <p> 081 * This method will have no effect if {@link #accept(String) a custom Accept header} is specified. 082 * </p> 083 * 084 * @see #accept(String) 085 * @see #encoded(EncodingEnum) 086 */ 087 T encodedXml(); 088 089 /** 090 * Set a HTTP header not explicitly defined in FHIR but commonly used in real-world scenarios. One 091 * important example is to set the Authorization header (e.g. Basic Auth or OAuth2-based Bearer auth), 092 * which tends to be cumbersome using {@link ca.uhn.fhir.rest.client.api.IClientInterceptor IClientInterceptors}, 093 * particularly when REST clients shall be reused and are thus supposed to remain stateless. 094 * <p>It is the responsibility of the caller to care for proper encoding of the header value, e.g. 095 * using Base64.</p> 096 * <p>This is a short-cut alternative to using a corresponding client interceptor</p> 097 * 098 * @param theHeaderName header name 099 * @param theHeaderValue header value 100 * @return 101 */ 102 T withAdditionalHeader(String theHeaderName, String theHeaderValue); 103 104 /** 105 * Actually execute the client operation 106 */ 107 Y execute(); 108 109 /** 110 * Explicitly specify a custom structure type to attempt to use when parsing the response. This 111 * is useful for invocations where the response is a Bundle/Parameters containing nested resources, 112 * and you want to use specific custom structures for those nested resources. 113 * <p> 114 * See <a href="https://jamesagnew.github.io/hapi-fhir/doc_extensions.html">Profiles and Extensions</a> for more information on using custom structures 115 * </p> 116 */ 117 T preferResponseType(Class<? extends IBaseResource> theType); 118 119 /** 120 * Explicitly specify a list of custom structure types to attempt to use (in order from most to 121 * least preferred) when parsing the response. This 122 * is useful for invocations where the response is a Bundle/Parameters containing nested resources, 123 * and you want to use specific custom structures for those nested resources. 124 * <p> 125 * See <a href="https://jamesagnew.github.io/hapi-fhir/doc_extensions.html">Profiles and Extensions</a> for more information on using custom structures 126 * </p> 127 */ 128 T preferResponseTypes(List<Class<? extends IBaseResource>> theTypes); 129 130 /** 131 * Request pretty-printed response via the <code>_pretty</code> parameter 132 */ 133 T prettyPrint(); 134 135 /** 136 * Request that the server modify the response using the <code>_summary</code> param 137 */ 138 T summaryMode(SummaryEnum theSummary); 139 140 /** 141 * Specifies a custom <code>Accept</code> header that should be supplied with the 142 * request. 143 * <p> 144 * Note that this method overrides any encoding preferences specified with 145 * {@link #encodedJson()} or {@link #encodedXml()}. It is generally easier to 146 * just use those methods if you simply want to request a specific FHIR encoding. 147 * </p> 148 * 149 * @param theHeaderValue The header value, e.g. "application/fhir+json". Constants such 150 * as {@link ca.uhn.fhir.rest.api.Constants#CT_FHIR_XML_NEW} and 151 * {@link ca.uhn.fhir.rest.api.Constants#CT_FHIR_JSON_NEW} may 152 * be useful. If set to <code>null</code> or an empty string, the 153 * default Accept header will be used. 154 * @see #encoded(EncodingEnum) 155 * @see #encodedJson() 156 * @see #encodedXml() 157 */ 158 T accept(String theHeaderValue); 159}