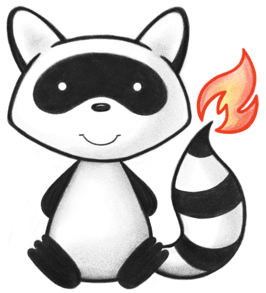
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.gclient; 021 022import ca.uhn.fhir.model.api.IQueryParameterType; 023import org.hl7.fhir.instance.model.api.IBase; 024import org.hl7.fhir.instance.model.api.IBaseParameters; 025 026public interface IOperationUntyped { 027 028 /** 029 * Use the given parameters resource as the input to the operation 030 * 031 * @param theParameters The parameters to use as input. May also be <code>null</code> if the operation 032 * does not require any input parameters. 033 */ 034 <T extends IBaseParameters> IOperationUntypedWithInputAndPartialOutput<T> withParameters(T theParameters); 035 036 /** 037 * The operation does not require any input parameters 038 * 039 * @param theOutputParameterType The type to use for the output parameters (this should be set to 040 * <code>Parameters.class</code> drawn from the version of the FHIR structures you are using) 041 */ 042 <T extends IBaseParameters> IOperationUntypedWithInput<T> withNoParameters(Class<T> theOutputParameterType); 043 044 /** 045 * Use chained method calls to construct a Parameters input. This form is a convenience 046 * in order to allow simple method chaining to be used to build up a parameters 047 * resource for the input of an operation without needing to manually construct one. 048 * <p> 049 * A sample invocation of this class could look like:<br/> 050 * <pre>Bundle bundle = client.operation() 051 * .onInstance(new IdType("Patient/A161443")) 052 * .named("everything") 053 * .withParameter(Parameters.class, "_count", new IntegerType(50)) 054 * .useHttpGet() 055 * .returnResourceType(Bundle.class) 056 * .execute(); 057 * </pre> 058 * </p> 059 * 060 * @param theParameterType The type to use for the output parameters (this should be set to 061 * <code>Parameters.class</code> drawn from the version of the FHIR structures you are using) 062 * @param theName The first parameter name 063 * @param theValue The first parameter value 064 */ 065 <T extends IBaseParameters> IOperationUntypedWithInputAndPartialOutput<T> withParameter( 066 Class<T> theParameterType, String theName, IBase theValue); 067 068 /** 069 * Use chained method calls to construct a Parameters input. This form is a convenience 070 * in order to allow simple method chaining to be used to build up a parameters 071 * resource for the input of an operation without needing to manually construct one. 072 * 073 * @param theParameterType The type to use for the output parameters (this should be set to 074 * <code>Parameters.class</code> drawn from the version of the FHIR structures you are using) 075 * @param theName The first parameter name 076 * @param theValue The first parameter value 077 */ 078 <T extends IBaseParameters> IOperationUntypedWithInputAndPartialOutput<T> withSearchParameter( 079 Class<T> theParameterType, String theName, IQueryParameterType theValue); 080}