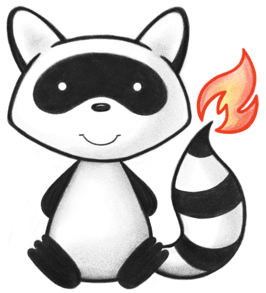
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.gclient; 021 022import ca.uhn.fhir.model.api.Include; 023import ca.uhn.fhir.rest.api.SearchStyleEnum; 024import ca.uhn.fhir.rest.api.SearchTotalModeEnum; 025import ca.uhn.fhir.rest.api.SortSpec; 026import ca.uhn.fhir.rest.param.DateRangeParam; 027import org.hl7.fhir.instance.model.api.IBaseBundle; 028 029import java.util.Collection; 030 031public interface IQuery<Y> extends IBaseQuery<IQuery<Y>>, IClientExecutable<IQuery<Y>, Y> { 032 033 /** 034 * {@inheritDoc} 035 */ 036 // This is here as an overridden method to allow mocking clients with Mockito to work 037 @Override 038 IQuery<Y> and(ICriterion<?> theCriterion); 039 040 /** 041 * Specifies the <code>_count</code> parameter, which indicates to the server how many resources should be returned 042 * on a single page. 043 * 044 * @since 1.4 045 */ 046 IQuery<Y> count(int theCount); 047 048 /** 049 * Specifies the <code>_offset</code> parameter, which indicates to the server the offset of the query. Use 050 * with {@link #count(int)}. 051 * 052 * This parameter is not part of the FHIR standard, all servers might not implement it. 053 * 054 * @since 5.2 055 */ 056 IQuery<Y> offset(int theOffset); 057 058 /** 059 * Add an "_include" specification or an "_include:recurse" specification. If you are using 060 * a constant from one of the built-in structures you can select whether you want recursive 061 * behaviour by using the following syntax: 062 * <ul> 063 * <li><b>Recurse:</b> <code>.include(Patient.INCLUDE_ORGANIZATION.asRecursive())</code> 064 * <li><b>No Recurse:</b> <code>.include(Patient.INCLUDE_ORGANIZATION.asNonRecursive())</code> 065 * </ul> 066 */ 067 IQuery<Y> include(Include theInclude); 068 069 /** 070 * Add a "_lastUpdated" specification 071 * 072 * @since HAPI FHIR 1.1 - Note that option was added to FHIR itself in DSTU2 073 */ 074 IQuery<Y> lastUpdated(DateRangeParam theLastUpdated); 075 076 /** 077 * Specifies the <code>_count</code> parameter, which indicates to the server how many resources should be returned 078 * on a single page. 079 * 080 * @deprecated This parameter is badly named, since FHIR calls this parameter "_count" and not "_limit". Use {@link #count(int)} instead (it also sets the _count parameter) 081 * @see #count(int) 082 */ 083 @Deprecated 084 IQuery<Y> limitTo(int theLimitTo); 085 086 /** 087 * Request that the client return the specified bundle type, e.g. <code>org.hl7.fhir.dstu2.model.Bundle.class</code> 088 * or <code>ca.uhn.fhir.model.dstu2.resource.Bundle.class</code> 089 */ 090 <B extends IBaseBundle> IQuery<B> returnBundle(Class<B> theClass); 091 092 /** 093 * Request that the server modify the response using the <code>_total</code> param 094 * 095 * THIS IS AN EXPERIMENTAL FEATURE - Use with caution, as it may be 096 * removed or modified in a future version. 097 */ 098 IQuery<Y> totalMode(SearchTotalModeEnum theTotalMode); 099 100 /** 101 * Add a "_revinclude" specification 102 * 103 * @since HAPI FHIR 1.0 - Note that option was added to FHIR itself in DSTU2 104 */ 105 IQuery<Y> revInclude(Include theIncludeTarget); 106 107 /** 108 * Adds a sort criteria 109 * 110 * @see #sort(SortSpec) for an alternate way of speciyfing sorts 111 */ 112 ISort<Y> sort(); 113 114 /** 115 * Adds a sort using a {@link SortSpec} object 116 * 117 * @see #sort() for an alternate way of speciyfing sorts 118 */ 119 IQuery<Y> sort(SortSpec theSortSpec); 120 121 /** 122 * Forces the query to perform the search using the given method (allowable methods are described in the 123 * <a href="http://www.hl7.org/fhir/search.html">FHIR Search Specification</a>) 124 * <p> 125 * This can be used to force the use of an HTTP POST instead of an HTTP GET 126 * </p> 127 * 128 * @see SearchStyleEnum 129 * @since 0.6 130 */ 131 IQuery<Y> usingStyle(SearchStyleEnum theStyle); 132 133 /** 134 * {@inheritDoc} 135 */ 136 // This is here as an overridden method to allow mocking clients with Mockito to work 137 @Override 138 IQuery<Y> where(ICriterion<?> theCriterion); 139 140 /** 141 * Matches any of the profiles given as argument. This would result in an OR search for resources matching one or more profiles. 142 * To do an AND search, make multiple calls to {@link #withProfile(String)}. 143 * 144 * @param theProfileUris The URIs of a given profile to search for resources which match. 145 */ 146 IQuery<Y> withAnyProfile(Collection<String> theProfileUris); 147 148 IQuery<Y> withIdAndCompartment(String theResourceId, String theCompartmentName); 149 150 /** 151 * Match only resources where the resource has the given profile declaration. This parameter corresponds to 152 * the <code>_profile</code> URL parameter. 153 * 154 * @param theProfileUri The URI of a given profile to search for resources which match 155 */ 156 IQuery<Y> withProfile(String theProfileUri); 157 158 /** 159 * Match only resources where the resource has the given security tag. This parameter corresponds to 160 * the <code>_security</code> URL parameter. 161 * 162 * @param theSystem The tag code system, or <code>null</code> to match any code system (this may not be supported on all servers) 163 * @param theCode The tag code. Must not be <code>null</code> or empty. 164 */ 165 IQuery<Y> withSecurity(String theSystem, String theCode); 166 167 /** 168 * Match only resources where the resource has the given tag. This parameter corresponds to 169 * the <code>_tag</code> URL parameter. 170 * 171 * @param theSystem The tag code system, or <code>null</code> to match any code system (this may not be supported on all servers) 172 * @param theCode The tag code. Must not be <code>null</code> or empty. 173 */ 174 IQuery<Y> withTag(String theSystem, String theCode); 175 176 // Y execute(); 177 178}