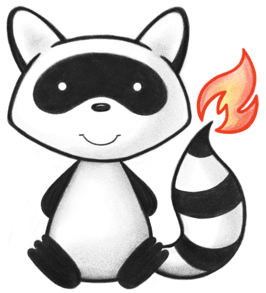
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.gclient; 021 022import ca.uhn.fhir.rest.api.Constants; 023import org.hl7.fhir.instance.model.api.IPrimitiveType; 024 025import java.util.Arrays; 026import java.util.List; 027 028/** 029 * 030 * @author james 031 * 032 */ 033public class StringClientParam extends BaseClientParam implements IParam { 034 035 private final String myParamName; 036 037 public StringClientParam(String theParamName) { 038 myParamName = theParamName; 039 } 040 041 @Override 042 public String getParamName() { 043 return myParamName; 044 } 045 046 /** 047 * The string matches the given value (servers will often, but are not required to) implement this as a left match, 048 * meaning that a value of "smi" would match "smi" and "smith". 049 */ 050 public IStringMatch matches() { 051 return new StringMatches(); 052 } 053 054 /** 055 * The string matches exactly the given value 056 */ 057 public IStringMatch matchesExactly() { 058 return new StringExactly(); 059 } 060 061 /** 062 * The string contains given value 063 */ 064 public IStringMatch contains() { 065 return new StringContains(); 066 } 067 068 public interface IStringMatch { 069 070 /** 071 * Requests that resources be returned which match the given value 072 */ 073 ICriterion<StringClientParam> value(String theValue); 074 075 /** 076 * Requests that resources be returned which match ANY of the given values (this is an OR search, not an AND search). Note that to 077 * specify an AND search, simply add a subsequent {@link IQuery#where(ICriterion) where} criteria with the same 078 * parameter. 079 */ 080 ICriterion<StringClientParam> values(List<String> theValues); 081 082 /** 083 * Requests that resources be returned which match the given value 084 */ 085 ICriterion<StringClientParam> value(IPrimitiveType<String> theValue); 086 087 /** 088 * Requests that resources be returned which match ANY of the given values (this is an OR search, not an AND search). Note that to 089 * specify an AND search, simply add a subsequent {@link IQuery#where(ICriterion) where} criteria with the same 090 * parameter. 091 */ 092 ICriterion<?> values(String... theValues); 093 } 094 095 private class StringExactly implements IStringMatch { 096 @Override 097 public ICriterion<StringClientParam> value(String theValue) { 098 return new StringCriterion<>(getParamName() + Constants.PARAMQUALIFIER_STRING_EXACT, theValue); 099 } 100 101 @Override 102 public ICriterion<StringClientParam> value(IPrimitiveType<String> theValue) { 103 return new StringCriterion<>(getParamName() + Constants.PARAMQUALIFIER_STRING_EXACT, theValue.getValue()); 104 } 105 106 @Override 107 public ICriterion<StringClientParam> values(List<String> theValue) { 108 return new StringCriterion<>(getParamName() + Constants.PARAMQUALIFIER_STRING_EXACT, theValue); 109 } 110 111 @Override 112 public ICriterion<?> values(String... theValues) { 113 return new StringCriterion<StringClientParam>( 114 getParamName() + Constants.PARAMQUALIFIER_STRING_EXACT, Arrays.asList(theValues)); 115 } 116 } 117 118 private class StringContains implements IStringMatch { 119 @Override 120 public ICriterion<StringClientParam> value(String theValue) { 121 return new StringCriterion<>(getParamName() + Constants.PARAMQUALIFIER_STRING_CONTAINS, theValue); 122 } 123 124 @Override 125 public ICriterion<StringClientParam> value(IPrimitiveType<String> theValue) { 126 return new StringCriterion<>( 127 getParamName() + Constants.PARAMQUALIFIER_STRING_CONTAINS, theValue.getValue()); 128 } 129 130 @Override 131 public ICriterion<StringClientParam> values(List<String> theValue) { 132 return new StringCriterion<>(getParamName() + Constants.PARAMQUALIFIER_STRING_CONTAINS, theValue); 133 } 134 135 @Override 136 public ICriterion<?> values(String... theValues) { 137 return new StringCriterion<StringClientParam>( 138 getParamName() + Constants.PARAMQUALIFIER_STRING_CONTAINS, Arrays.asList(theValues)); 139 } 140 } 141 142 private class StringMatches implements IStringMatch { 143 @Override 144 public ICriterion<StringClientParam> value(String theValue) { 145 return new StringCriterion<>(getParamName(), theValue); 146 } 147 148 @Override 149 public ICriterion<StringClientParam> value(IPrimitiveType<String> theValue) { 150 return new StringCriterion<>(getParamName(), theValue.getValue()); 151 } 152 153 @Override 154 public ICriterion<StringClientParam> values(List<String> theValue) { 155 return new StringCriterion<>(getParamName(), theValue); 156 } 157 158 @Override 159 public ICriterion<?> values(String... theValues) { 160 return new StringCriterion<StringClientParam>(getParamName(), Arrays.asList(theValues)); 161 } 162 } 163}