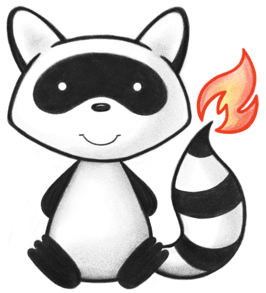
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.gclient; 021 022import ca.uhn.fhir.model.primitive.StringDt; 023import ca.uhn.fhir.util.CoverageIgnore; 024 025import java.util.Arrays; 026import java.util.List; 027 028/** 029 * 030 */ 031public class UriClientParam extends BaseClientParam implements IParam { 032 033 // TODO: handle :above and :below 034 035 private final String myParamName; 036 037 public UriClientParam(String theParamName) { 038 myParamName = theParamName; 039 } 040 041 @Override 042 public String getParamName() { 043 return myParamName; 044 } 045 046 /** 047 * The string matches the given value (servers will often, but are not required to) implement this as a left match, meaning that a value of "smi" would match "smi" and "smith". 048 * @param theValue THIS PARAMETER DOES NOT DO ANYTHING - This method was added by accident 049 * 050 * @deprecated theValue does not do anything, use {@link #matches()} instead 051 */ 052 @CoverageIgnore 053 @Deprecated 054 public IUriMatch matches(String theValue) { 055 return new UriMatches(); 056 } 057 058 /** 059 * The string matches the given value (servers will often, but are not required to) implement this as a left match, meaning that a value of "smi" would match "smi" and "smith". 060 */ 061 public IUriMatch matches() { 062 return new UriMatches(); 063 } 064 065 public interface IUriMatch { 066 067 /** 068 * Requests that resources be returned which match the given value 069 */ 070 ICriterion<UriClientParam> value(String theValue); 071 072 /** 073 * Requests that resources be returned which match ANY of the given values (this is an OR search). Note that to specify an AND search, simply add a subsequent {@link IQuery#where(ICriterion) 074 * where} criteria with the same parameter. 075 */ 076 ICriterion<UriClientParam> values(List<String> theValues); 077 078 /** 079 * Requests that resources be returned which match the given value 080 */ 081 ICriterion<UriClientParam> value(StringDt theValue); 082 083 /** 084 * Requests that resources be returned which match ANY of the given values (this is an OR search). Note that to specify an AND search, simply add a subsequent {@link IQuery#where(ICriterion) 085 * where} criteria with the same parameter. 086 */ 087 ICriterion<?> values(String... theValues); 088 } 089 090 private class UriMatches implements IUriMatch { 091 @Override 092 public ICriterion<UriClientParam> value(String theValue) { 093 return new StringCriterion<UriClientParam>(getParamName(), theValue); 094 } 095 096 @Override 097 public ICriterion<UriClientParam> value(StringDt theValue) { 098 return new StringCriterion<UriClientParam>(getParamName(), theValue.getValue()); 099 } 100 101 @Override 102 public ICriterion<UriClientParam> values(List<String> theValue) { 103 return new StringCriterion<UriClientParam>(getParamName(), theValue); 104 } 105 106 @Override 107 public ICriterion<?> values(String... theValues) { 108 return new StringCriterion<UriClientParam>(getParamName(), Arrays.asList(theValues)); 109 } 110 } 111}