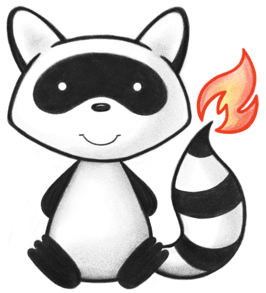
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.param; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.model.api.IQueryParameterAnd; 024import ca.uhn.fhir.model.api.IQueryParameterOr; 025import ca.uhn.fhir.rest.api.QualifiedParamList; 026import ca.uhn.fhir.rest.server.exceptions.InvalidRequestException; 027 028import java.util.ArrayList; 029import java.util.List; 030 031public abstract class BaseAndListParam<T extends IQueryParameterOr<?>> implements IQueryParameterAnd<T> { 032 033 private List<T> myValues = new ArrayList<>(); 034 035 public abstract BaseAndListParam<T> addAnd(T theValue); 036 037 public BaseAndListParam<T> addValue(T theValue) { 038 myValues.add(theValue); 039 return this; 040 } 041 042 @Override 043 public List<T> getValuesAsQueryTokens() { 044 return myValues; 045 } 046 047 abstract T newInstance(); 048 049 @Override 050 public void setValuesAsQueryTokens( 051 FhirContext theContext, String theParamName, List<QualifiedParamList> theParameters) 052 throws InvalidRequestException { 053 myValues.clear(); 054 for (QualifiedParamList nextParam : theParameters) { 055 T nextList = newInstance(); 056 nextList.setValuesAsQueryTokens(theContext, theParamName, nextParam); 057 myValues.add(nextList); 058 } 059 } 060 061 @Override 062 public String toString() { 063 return myValues.toString(); 064 } 065 066 /** 067 * Returns the number of AND parameters 068 */ 069 public int size() { 070 return myValues.size(); 071 } 072}