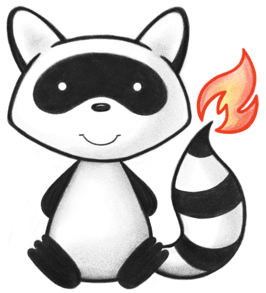
001/*- 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.param; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.i18n.Msg; 024import ca.uhn.fhir.model.api.IQueryParameterType; 025import ca.uhn.fhir.rest.api.Constants; 026import ca.uhn.fhir.rest.server.exceptions.InvalidRequestException; 027 028import static org.apache.commons.lang3.StringUtils.isNotBlank; 029 030/** 031 * Base class for RESTful operation parameter types 032 */ 033public abstract class BaseParam implements IQueryParameterType { 034 035 private Boolean myMissing; 036 037 abstract String doGetQueryParameterQualifier(); 038 039 abstract String doGetValueAsQueryToken(FhirContext theContext); 040 041 abstract void doSetValueAsQueryToken( 042 FhirContext theContext, String theParamName, String theQualifier, String theValue); 043 044 /** 045 * If set to non-null value, indicates that this parameter has been populated with a "[name]:missing=true" or "[name]:missing=false" vale instead of a normal value 046 */ 047 @Override 048 public Boolean getMissing() { 049 return myMissing; 050 } 051 052 @Override 053 public final String getQueryParameterQualifier() { 054 if (myMissing != null) { 055 return Constants.PARAMQUALIFIER_MISSING; 056 } 057 return doGetQueryParameterQualifier(); 058 } 059 060 @Override 061 public final String getValueAsQueryToken(FhirContext theContext) { 062 if (myMissing != null) { 063 return myMissing ? Constants.PARAMQUALIFIER_MISSING_TRUE : Constants.PARAMQUALIFIER_MISSING_FALSE; 064 } 065 return doGetValueAsQueryToken(theContext); 066 } 067 068 /** 069 * Does this parameter type support chained parameters (only reference should return <code>true</code> for this) 070 */ 071 protected boolean isSupportsChain() { 072 return false; 073 } 074 075 /** 076 * If set to non-null value, indicates that this parameter has been populated 077 * with a "[name]:missing=true" or "[name]:missing=false" value instead of a 078 * normal value 079 * 080 * @return Returns a reference to <code>this</code> for easier method chaining 081 */ 082 @Override 083 public BaseParam setMissing(Boolean theMissing) { 084 myMissing = theMissing; 085 return this; 086 } 087 088 @Override 089 public final void setValueAsQueryToken( 090 FhirContext theContext, String theParamName, String theQualifier, String theValue) { 091 if (Constants.PARAMQUALIFIER_MISSING.equals(theQualifier)) { 092 myMissing = "true".equals(theValue); 093 doSetValueAsQueryToken(theContext, theParamName, null, null); 094 } else { 095 if (isNotBlank(theQualifier) && theQualifier.charAt(0) == '.') { 096 if (!isSupportsChain()) { 097 String msg = theContext 098 .getLocalizer() 099 .getMessage( 100 BaseParam.class, "chainNotSupported", theParamName + theQualifier, theQualifier); 101 throw new InvalidRequestException(Msg.code(1935) + msg); 102 } 103 } 104 105 myMissing = null; 106 doSetValueAsQueryToken(theContext, theParamName, theQualifier, theValue); 107 } 108 } 109}