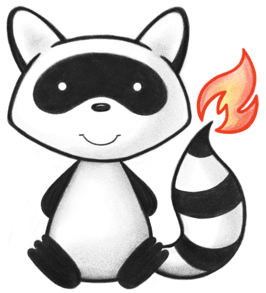
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.param; 021 022import ca.uhn.fhir.context.ConfigurationException; 023import ca.uhn.fhir.context.FhirContext; 024import ca.uhn.fhir.i18n.Msg; 025import ca.uhn.fhir.model.api.IQueryParameterType; 026import ca.uhn.fhir.rest.server.exceptions.InvalidRequestException; 027import org.apache.commons.lang3.Validate; 028import org.apache.commons.lang3.builder.ToStringBuilder; 029import org.apache.commons.lang3.builder.ToStringStyle; 030 031import java.util.Arrays; 032import java.util.Collections; 033import java.util.List; 034 035import static org.apache.commons.lang3.StringUtils.isBlank; 036 037public class CompositeParam<A extends IQueryParameterType, B extends IQueryParameterType> extends BaseParam 038 implements IQueryParameterType { 039 040 private A myLeftType; 041 private B myRightType; 042 043 public CompositeParam(A theLeftInstance, B theRightInstance) { 044 myLeftType = theLeftInstance; 045 myRightType = theRightInstance; 046 } 047 048 public CompositeParam(Class<A> theLeftType, Class<B> theRightType) { 049 Validate.notNull(theLeftType); 050 Validate.notNull(theRightType); 051 try { 052 myLeftType = theLeftType.newInstance(); 053 } catch (InstantiationException e) { 054 throw new ConfigurationException(Msg.code(1943) + "Failed to instantiate type: " + myLeftType, e); 055 } catch (IllegalAccessException e) { 056 throw new ConfigurationException(Msg.code(1944) + "Failed to instantiate type: " + myLeftType, e); 057 } 058 try { 059 myRightType = theRightType.newInstance(); 060 } catch (InstantiationException e) { 061 throw new ConfigurationException(Msg.code(1945) + "Failed to instantiate type: " + myRightType, e); 062 } catch (IllegalAccessException e) { 063 throw new ConfigurationException(Msg.code(1946) + "Failed to instantiate type: " + myRightType, e); 064 } 065 } 066 067 @Override 068 String doGetQueryParameterQualifier() { 069 return null; 070 } 071 072 @Override 073 String doGetValueAsQueryToken(FhirContext theContext) { 074 StringBuilder b = new StringBuilder(); 075 if (myLeftType != null) { 076 b.append(myLeftType.getValueAsQueryToken(theContext)); 077 } 078 b.append('$'); 079 if (myRightType != null) { 080 b.append(myRightType.getValueAsQueryToken(theContext)); 081 } 082 return b.toString(); 083 } 084 085 @Override 086 void doSetValueAsQueryToken(FhirContext theContext, String theParamName, String theQualifier, String theValue) { 087 if (isBlank(theValue)) { 088 myLeftType.setValueAsQueryToken(theContext, theParamName, theQualifier, ""); 089 myRightType.setValueAsQueryToken(theContext, theParamName, theQualifier, ""); 090 } else { 091 List<String> parts = ParameterUtil.splitParameterString(theValue, '$', false); 092 if (parts.size() > 2) { 093 throw new InvalidRequestException(Msg.code(1947) 094 + "Invalid value for composite parameter (only one '$' is valid for this parameter, others must be escaped). Value was: " 095 + theValue); 096 } 097 myLeftType.setValueAsQueryToken(theContext, theParamName, theQualifier, parts.get(0)); 098 if (parts.size() > 1) { 099 myRightType.setValueAsQueryToken(theContext, theParamName, theQualifier, parts.get(1)); 100 } 101 } 102 } 103 104 /** 105 * @return Returns the left value for this parameter (the first of two parameters in this composite) 106 */ 107 public A getLeftValue() { 108 return myLeftType; 109 } 110 111 /** 112 * @return Returns the right value for this parameter (the second of two parameters in this composite) 113 */ 114 public B getRightValue() { 115 return myRightType; 116 } 117 118 /** 119 * Get the values of the subcomponents, in order. 120 */ 121 public List<IQueryParameterType> getValues() { 122 return Collections.unmodifiableList(Arrays.asList(myLeftType, myRightType)); 123 } 124 125 @Override 126 public String toString() { 127 ToStringBuilder b = new ToStringBuilder(this, ToStringStyle.SHORT_PREFIX_STYLE); 128 b.append("myLeftType", getLeftValue()); 129 b.append("myRightType", getRightValue()); 130 return b.toString(); 131 } 132}