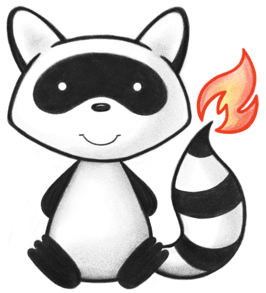
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.param; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.i18n.Msg; 024import ca.uhn.fhir.model.api.IQueryParameterOr; 025import ca.uhn.fhir.model.api.TemporalPrecisionEnum; 026import ca.uhn.fhir.model.primitive.BaseDateTimeDt; 027import ca.uhn.fhir.model.primitive.DateDt; 028import ca.uhn.fhir.model.primitive.DateTimeDt; 029import ca.uhn.fhir.rest.api.QualifiedParamList; 030import ca.uhn.fhir.rest.server.exceptions.InvalidRequestException; 031import ca.uhn.fhir.util.ValidateUtil; 032import org.apache.commons.lang3.builder.ToStringBuilder; 033import org.apache.commons.lang3.builder.ToStringStyle; 034import org.hl7.fhir.instance.model.api.IPrimitiveType; 035 036import java.util.Collections; 037import java.util.Date; 038import java.util.List; 039import java.util.Objects; 040 041import static org.apache.commons.lang3.StringUtils.isNotBlank; 042 043public class DateParam extends BaseParamWithPrefix<DateParam> 044 implements /*IQueryParameterType , */ IQueryParameterOr<DateParam> { 045 046 private static final long serialVersionUID = 1L; 047 048 private final DateParamDateTimeHolder myValue = new DateParamDateTimeHolder(); 049 050 /** 051 * Constructor 052 */ 053 public DateParam() { 054 super(); 055 } 056 057 /** 058 * Constructor 059 */ 060 public DateParam(ParamPrefixEnum thePrefix, Date theDate) { 061 setPrefix(thePrefix); 062 setValue(theDate); 063 } 064 065 /** 066 * Constructor 067 */ 068 public DateParam(ParamPrefixEnum thePrefix, DateTimeDt theDate) { 069 setPrefix(thePrefix); 070 myValue.setValueAsString(theDate != null ? theDate.getValueAsString() : null); 071 } 072 073 /** 074 * Constructor 075 */ 076 public DateParam(ParamPrefixEnum thePrefix, IPrimitiveType<Date> theDate) { 077 setPrefix(thePrefix); 078 myValue.setValueAsString(theDate != null ? theDate.getValueAsString() : null); 079 } 080 081 /** 082 * Constructor 083 */ 084 public DateParam(ParamPrefixEnum thePrefix, long theDate) { 085 ValidateUtil.isGreaterThan(theDate, 0, "theDate must not be 0 or negative"); 086 setPrefix(thePrefix); 087 setValue(new Date(theDate)); 088 } 089 090 /** 091 * Constructor 092 */ 093 public DateParam(ParamPrefixEnum thePrefix, String theDate) { 094 setPrefix(thePrefix); 095 setValueAsString(theDate); 096 } 097 098 /** 099 * Constructor which takes a complete [qualifier]{date} string. 100 * 101 * @param theString 102 * The string 103 */ 104 public DateParam(String theString) { 105 setValueAsQueryToken(null, null, null, theString); 106 } 107 108 @Override 109 String doGetQueryParameterQualifier() { 110 return null; 111 } 112 113 @Override 114 String doGetValueAsQueryToken(FhirContext theContext) { 115 StringBuilder b = new StringBuilder(); 116 if (getPrefix() != null) { 117 b.append(ParameterUtil.escapeWithDefault(getPrefix().getValue())); 118 } 119 120 b.append(ParameterUtil.escapeWithDefault(myValue.getValueAsString())); 121 122 return b.toString(); 123 } 124 125 @Override 126 void doSetValueAsQueryToken(FhirContext theContext, String theParamName, String theQualifier, String theValue) { 127 setValueAsString(theValue); 128 } 129 130 public TemporalPrecisionEnum getPrecision() { 131 return myValue.getPrecision(); 132 } 133 134 public Date getValue() { 135 return myValue.getValue(); 136 } 137 138 public String getValueAsString() { 139 return myValue.getValueAsString(); 140 } 141 142 @Override 143 public List<DateParam> getValuesAsQueryTokens() { 144 return Collections.singletonList(this); 145 } 146 147 /** 148 * Returns <code>true</code> if no date/time is specified. Note that this method does not check the comparator, so a 149 * QualifiedDateParam with only a comparator and no date/time is considered empty. 150 */ 151 public boolean isEmpty() { 152 return myValue.isEmpty(); 153 } 154 155 /** 156 * Sets the value of the param to the given date (sets to the {@link TemporalPrecisionEnum#MILLI millisecond} 157 * precision, and will be encoded using the system local time zone). 158 */ 159 public DateParam setValue(Date theValue) { 160 myValue.setValue(theValue, TemporalPrecisionEnum.MILLI); 161 return this; 162 } 163 164 /** 165 * Sets the value using a FHIR Date type, such as a {@link DateDt}, or a DateTimeType. 166 */ 167 public void setValue(IPrimitiveType<Date> theValue) { 168 if (theValue != null) { 169 myValue.setValueAsString(theValue.getValueAsString()); 170 } else { 171 myValue.setValue(null); 172 } 173 } 174 175 /** 176 * Accepts values with or without a prefix (e.g. <code>gt2011-01-01</code> and <code>2011-01-01</code>). 177 * If no prefix is provided in the given value, the {@link #getPrefix() existing prefix} is preserved 178 */ 179 public void setValueAsString(String theDate) { 180 if (isNotBlank(theDate)) { 181 ParamPrefixEnum existingPrefix = getPrefix(); 182 myValue.setValueAsString(super.extractPrefixAndReturnRest(theDate)); 183 if (getPrefix() == null) { 184 setPrefix(existingPrefix); 185 } 186 } else { 187 myValue.setValue(null); 188 } 189 } 190 191 @Override 192 public void setValuesAsQueryTokens(FhirContext theContext, String theParamName, QualifiedParamList theParameters) { 193 setMissing(null); 194 setPrefix(null); 195 setValueAsString(null); 196 197 if (theParameters.size() == 1) { 198 setValueAsString(theParameters.get(0)); 199 } else if (theParameters.size() > 1) { 200 throw new InvalidRequestException(Msg.code(1939) 201 + "This server does not support multi-valued dates for this parameter: " + theParameters); 202 } 203 } 204 205 @Override 206 public boolean equals(Object obj) { 207 if (obj == this) { 208 return true; 209 } 210 if (!(obj instanceof DateParam)) { 211 return false; 212 } 213 DateParam other = (DateParam) obj; 214 return Objects.equals(getValue(), other.getValue()) && Objects.equals(getPrefix(), other.getPrefix()); 215 } 216 217 @Override 218 public int hashCode() { 219 return Objects.hash(getValue(), getPrefix()); 220 } 221 222 @Override 223 public String toString() { 224 ToStringBuilder b = new ToStringBuilder(this, ToStringStyle.SHORT_PREFIX_STYLE); 225 b.append("prefix", getPrefix()); 226 b.append("value", getValueAsString()); 227 return b.build(); 228 } 229 230 public static class DateParamDateTimeHolder extends BaseDateTimeDt { 231 232 /** 233 * Constructor 234 */ 235 // LEAVE THIS AS PUBLIC!! 236 @SuppressWarnings("WeakerAccess") 237 public DateParamDateTimeHolder() { 238 super(); 239 } 240 241 @Override 242 protected TemporalPrecisionEnum getDefaultPrecisionForDatatype() { 243 return TemporalPrecisionEnum.SECOND; 244 } 245 246 @Override 247 protected boolean isPrecisionAllowed(TemporalPrecisionEnum thePrecision) { 248 return true; 249 } 250 } 251}