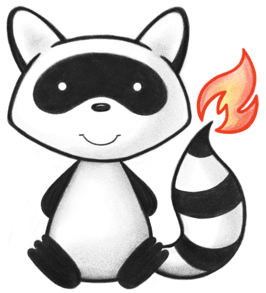
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.param; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.i18n.Msg; 024import ca.uhn.fhir.model.api.IQueryParameterType; 025import ca.uhn.fhir.rest.api.Constants; 026import ca.uhn.fhir.rest.server.exceptions.InvalidRequestException; 027 028import static org.apache.commons.lang3.StringUtils.defaultString; 029 030/** 031 * Implementation of the _has method parameter 032 */ 033public class HasParam extends BaseParam implements IQueryParameterType { 034 035 private static final long serialVersionUID = 1L; 036 037 private String myReferenceFieldName; 038 private String myParameterName; 039 private String myParameterValue; 040 private String myTargetResourceType; 041 042 public HasParam() { 043 super(); 044 } 045 046 public HasParam( 047 String theTargetResourceType, 048 String theReferenceFieldName, 049 String theParameterName, 050 String theParameterValue) { 051 this(); 052 myTargetResourceType = theTargetResourceType; 053 myReferenceFieldName = theReferenceFieldName; 054 myParameterName = theParameterName; 055 myParameterValue = theParameterValue; 056 } 057 058 @Override 059 String doGetQueryParameterQualifier() { 060 return ':' + myTargetResourceType + ':' + myReferenceFieldName + ':' + myParameterName; 061 } 062 063 @Override 064 String doGetValueAsQueryToken(FhirContext theContext) { 065 return myParameterValue; 066 } 067 068 @Override 069 void doSetValueAsQueryToken(FhirContext theContext, String theParamName, String theQualifier, String theValue) { 070 String qualifier = defaultString(theQualifier); 071 if (!qualifier.startsWith(":")) { 072 throwInvalidSyntaxException(Constants.PARAM_HAS + qualifier); 073 } 074 int colonIndex0 = qualifier.indexOf(':', 1); 075 validateColon(qualifier, colonIndex0); 076 int colonIndex1 = qualifier.indexOf(':', colonIndex0 + 1); 077 validateColon(qualifier, colonIndex1); 078 079 myTargetResourceType = qualifier.substring(1, colonIndex0); 080 myReferenceFieldName = qualifier.substring(colonIndex0 + 1, colonIndex1); 081 myParameterName = qualifier.substring(colonIndex1 + 1); 082 myParameterValue = theValue; 083 } 084 085 public String getReferenceFieldName() { 086 return myReferenceFieldName; 087 } 088 089 public String getParameterName() { 090 return myParameterName; 091 } 092 093 public String getParameterValue() { 094 return myParameterValue; 095 } 096 097 public String getTargetResourceType() { 098 return myTargetResourceType; 099 } 100 101 private static void validateColon(String theParameterName, int colonIndex) { 102 if (colonIndex == -1) { 103 throwInvalidSyntaxException(theParameterName); 104 } 105 } 106 107 private static void throwInvalidSyntaxException(String theParameterName) { 108 throw new InvalidRequestException(Msg.code(1942) + "Invalid _has parameter syntax: " + theParameterName); 109 } 110}