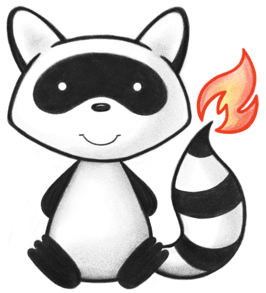
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.param; 021 022import ca.uhn.fhir.i18n.Msg; 023import ca.uhn.fhir.model.api.ICompositeDatatype; 024import ca.uhn.fhir.model.api.IElement; 025import ca.uhn.fhir.model.api.IQueryParameterType; 026import ca.uhn.fhir.model.api.annotation.Child; 027import ca.uhn.fhir.model.api.annotation.Description; 028import ca.uhn.fhir.model.base.composite.BaseCodingDt; 029import ca.uhn.fhir.model.base.composite.BaseResourceReferenceDt; 030import ca.uhn.fhir.model.primitive.BooleanDt; 031import ca.uhn.fhir.model.primitive.CodeDt; 032import ca.uhn.fhir.model.primitive.StringDt; 033import ca.uhn.fhir.model.primitive.UriDt; 034import ca.uhn.fhir.util.CoverageIgnore; 035 036import java.util.List; 037 038@CoverageIgnore 039public class InternalCodingDt extends BaseCodingDt implements ICompositeDatatype { 040 041 private static final long serialVersionUID = 993056016725918652L; 042 043 /** 044 * Constructor 045 */ 046 public InternalCodingDt() { 047 super(); 048 } 049 050 /** 051 * Creates a new Coding with the given system and code 052 */ 053 public InternalCodingDt(String theSystem, String theCode) { 054 setSystem(theSystem); 055 setCode(theCode); 056 } 057 058 @Child(name = "system", type = UriDt.class, order = 0, min = 0, max = 1) 059 @Description( 060 shortDefinition = "Identity of the terminology system", 061 formalDefinition = 062 "The identification of the code system that defines the meaning of the symbol in the code.") 063 private UriDt mySystem; 064 065 @Child(name = "version", type = StringDt.class, order = 1, min = 0, max = 1) 066 @Description( 067 shortDefinition = "Version of the system - if relevant", 068 formalDefinition = 069 "The version of the code system which was used when choosing this code. Note that a well-maintained code system does not need the version reported, because the meaning of codes is consistent across versions. However this cannot consistently be assured. and When the meaning is not guaranteed to be consistent, the version SHOULD be exchanged") 070 private StringDt myVersion; 071 072 @Child(name = "code", type = CodeDt.class, order = 2, min = 0, max = 1) 073 @Description( 074 shortDefinition = "Symbol in syntax defined by the system", 075 formalDefinition = 076 "A symbol in syntax defined by the system. The symbol may be a predefined code or an expression in a syntax defined by the coding system (e.g. post-coordination)") 077 private CodeDt myCode; 078 079 @Child(name = "display", type = StringDt.class, order = 3, min = 0, max = 1) 080 @Description( 081 shortDefinition = "Representation defined by the system", 082 formalDefinition = 083 "A representation of the meaning of the code in the system, following the rules of the system.") 084 private StringDt myDisplay; 085 086 @Child(name = "primary", type = BooleanDt.class, order = 4, min = 0, max = 1) 087 @Description( 088 shortDefinition = "If this code was chosen directly by the user", 089 formalDefinition = 090 "Indicates that this code was chosen by a user directly - i.e. off a pick list of available items (codes or displays)") 091 private BooleanDt myPrimary; 092 093 @Override 094 public boolean isEmpty() { 095 return super.isBaseEmpty() 096 && ca.uhn.fhir.util.ElementUtil.isEmpty(mySystem, myVersion, myCode, myDisplay, myPrimary); 097 } 098 099 @Deprecated // override deprecated method 100 @Override 101 public <T extends IElement> List<T> getAllPopulatedChildElementsOfType(Class<T> theType) { 102 return ca.uhn.fhir.util.ElementUtil.allPopulatedChildElements( 103 theType, mySystem, myVersion, myCode, myDisplay, myPrimary); 104 } 105 106 /** 107 * Gets the value(s) for <b>system</b> (Identity of the terminology system). creating it if it does not exist. Will not return <code>null</code>. 108 * 109 * <p> 110 * <b>Definition:</b> The identification of the code system that defines the meaning of the symbol in the code. 111 * </p> 112 */ 113 @Override 114 public UriDt getSystemElement() { 115 if (mySystem == null) { 116 mySystem = new UriDt(); 117 } 118 return mySystem; 119 } 120 121 /** 122 * Sets the value(s) for <b>system</b> (Identity of the terminology system) 123 * 124 * <p> 125 * <b>Definition:</b> The identification of the code system that defines the meaning of the symbol in the code. 126 * </p> 127 */ 128 public InternalCodingDt setSystem(UriDt theValue) { 129 mySystem = theValue; 130 return this; 131 } 132 133 /** 134 * Sets the value for <b>system</b> (Identity of the terminology system) 135 * 136 * <p> 137 * <b>Definition:</b> The identification of the code system that defines the meaning of the symbol in the code. 138 * </p> 139 */ 140 @Override 141 public InternalCodingDt setSystem(String theUri) { 142 mySystem = new UriDt(theUri); 143 return this; 144 } 145 146 /** 147 * Gets the value(s) for <b>version</b> (Version of the system - if relevant). creating it if it does not exist. Will not return <code>null</code>. 148 * 149 * <p> 150 * <b>Definition:</b> The version of the code system which was used when choosing this code. Note that a well-maintained code system does not need the version reported, because the meaning of codes 151 * is consistent across versions. However this cannot consistently be assured. and When the meaning is not guaranteed to be consistent, the version SHOULD be exchanged 152 * </p> 153 */ 154 @Override 155 public StringDt getVersionElement() { 156 if (myVersion == null) { 157 myVersion = new StringDt(); 158 } 159 return myVersion; 160 } 161 162 @Override 163 public BooleanDt getUserSelectedElement() { 164 return new BooleanDt(); 165 } 166 167 /** 168 * Legacy name for {@link #getVersionElement()} 169 */ 170 @Deprecated(since = "7.0.0") 171 public StringDt getVersion() { 172 return getVersionElement(); 173 } 174 175 /** 176 * Sets the value(s) for <b>version</b> (Version of the system - if relevant) 177 * 178 * <p> 179 * <b>Definition:</b> The version of the code system which was used when choosing this code. Note that a well-maintained code system does not need the version reported, because the meaning of codes 180 * is consistent across versions. However this cannot consistently be assured. and When the meaning is not guaranteed to be consistent, the version SHOULD be exchanged 181 * </p> 182 */ 183 public InternalCodingDt setVersion(StringDt theValue) { 184 myVersion = theValue; 185 return this; 186 } 187 188 /** 189 * Sets the value for <b>version</b> (Version of the system - if relevant) 190 * 191 * <p> 192 * <b>Definition:</b> The version of the code system which was used when choosing this code. Note that a well-maintained code system does not need the version reported, because the meaning of codes 193 * is consistent across versions. However this cannot consistently be assured. and When the meaning is not guaranteed to be consistent, the version SHOULD be exchanged 194 * </p> 195 */ 196 public InternalCodingDt setVersion(String theString) { 197 myVersion = new StringDt(theString); 198 return this; 199 } 200 201 /** 202 * Gets the value(s) for <b>code</b> (Symbol in syntax defined by the system). creating it if it does not exist. Will not return <code>null</code>. 203 * 204 * <p> 205 * <b>Definition:</b> A symbol in syntax defined by the system. The symbol may be a predefined code or an expression in a syntax defined by the coding system (e.g. post-coordination) 206 * </p> 207 */ 208 @Override 209 public CodeDt getCodeElement() { 210 if (myCode == null) { 211 myCode = new CodeDt(); 212 } 213 return myCode; 214 } 215 216 /** 217 * Sets the value(s) for <b>code</b> (Symbol in syntax defined by the system) 218 * 219 * <p> 220 * <b>Definition:</b> A symbol in syntax defined by the system. The symbol may be a predefined code or an expression in a syntax defined by the coding system (e.g. post-coordination) 221 * </p> 222 */ 223 public InternalCodingDt setCode(CodeDt theValue) { 224 myCode = theValue; 225 return this; 226 } 227 228 /** 229 * Sets the value for <b>code</b> (Symbol in syntax defined by the system) 230 * 231 * <p> 232 * <b>Definition:</b> A symbol in syntax defined by the system. The symbol may be a predefined code or an expression in a syntax defined by the coding system (e.g. post-coordination) 233 * </p> 234 */ 235 @Override 236 public InternalCodingDt setCode(String theCode) { 237 myCode = new CodeDt(theCode); 238 return this; 239 } 240 241 /** 242 * Gets the value(s) for <b>display</b> (Representation defined by the system). creating it if it does not exist. Will not return <code>null</code>. 243 * 244 * <p> 245 * <b>Definition:</b> A representation of the meaning of the code in the system, following the rules of the system. 246 * </p> 247 */ 248 public StringDt getDisplay() { 249 if (myDisplay == null) { 250 myDisplay = new StringDt(); 251 } 252 return myDisplay; 253 } 254 255 /** 256 * Sets the value(s) for <b>display</b> (Representation defined by the system) 257 * 258 * <p> 259 * <b>Definition:</b> A representation of the meaning of the code in the system, following the rules of the system. 260 * </p> 261 */ 262 public InternalCodingDt setDisplay(StringDt theValue) { 263 myDisplay = theValue; 264 return this; 265 } 266 267 /** 268 * Sets the value for <b>display</b> (Representation defined by the system) 269 * 270 * <p> 271 * <b>Definition:</b> A representation of the meaning of the code in the system, following the rules of the system. 272 * </p> 273 */ 274 @Override 275 public InternalCodingDt setDisplay(String theString) { 276 myDisplay = new StringDt(theString); 277 return this; 278 } 279 280 /** 281 * Gets the value(s) for <b>primary</b> (If this code was chosen directly by the user). creating it if it does not exist. Will not return <code>null</code>. 282 * 283 * <p> 284 * <b>Definition:</b> Indicates that this code was chosen by a user directly - i.e. off a pick list of available items (codes or displays) 285 * </p> 286 */ 287 public BooleanDt getPrimary() { 288 if (myPrimary == null) { 289 myPrimary = new BooleanDt(); 290 } 291 return myPrimary; 292 } 293 294 /** 295 * Sets the value(s) for <b>primary</b> (If this code was chosen directly by the user) 296 * 297 * <p> 298 * <b>Definition:</b> Indicates that this code was chosen by a user directly - i.e. off a pick list of available items (codes or displays) 299 * </p> 300 */ 301 public InternalCodingDt setPrimary(BooleanDt theValue) { 302 myPrimary = theValue; 303 return this; 304 } 305 306 /** 307 * Sets the value for <b>primary</b> (If this code was chosen directly by the user) 308 * 309 * <p> 310 * <b>Definition:</b> Indicates that this code was chosen by a user directly - i.e. off a pick list of available items (codes or displays) 311 * </p> 312 */ 313 public InternalCodingDt setPrimary(boolean theBoolean) { 314 myPrimary = new BooleanDt(theBoolean); 315 return this; 316 } 317 318 /** 319 * Gets the value(s) for <b>valueSet</b> (Set this coding was chosen from). creating it if it does not exist. Will not return <code>null</code>. 320 * 321 * <p> 322 * <b>Definition:</b> The set of possible coded values this coding was chosen from or constrained by 323 * </p> 324 */ 325 public BaseResourceReferenceDt getValueSet() { 326 throw new UnsupportedOperationException(Msg.code(1949)); 327 } 328 329 @Override 330 public StringDt getDisplayElement() { 331 return getDisplay(); 332 } 333 334 @Deprecated // override deprecated method 335 @Override 336 public Boolean getMissing() { 337 throw new UnsupportedOperationException(Msg.code(1950)); 338 } 339 340 @Deprecated // override deprecated method 341 @Override 342 public IQueryParameterType setMissing(Boolean theMissing) { 343 throw new UnsupportedOperationException(Msg.code(1951)); 344 } 345}