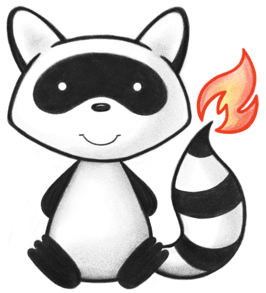
001/*- 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.param; 021 022import java.util.Collections; 023import java.util.HashMap; 024import java.util.Map; 025 026/** 027 * Comparator/qualifier for values used in REST params, such as {@link DateParam}, {@link NumberParam}, and 028 * {@link QuantityParam} 029 * 030 * @since 1.5 031 */ 032public enum ParamPrefixEnum { 033 034 /** 035 * Code Value: <b>eq</b> 036 * 037 * The actual value is equal to the given value 038 */ 039 APPROXIMATE("ap"), 040 041 /** 042 * Code Value: <b>eb</b> 043 * 044 * The range of the search value does overlap not with the range of the target value, and the range above the search value contains the range of the target value 045 */ 046 ENDS_BEFORE("eb"), 047 048 /** 049 * Code Value: <b>eq</b> 050 * 051 * The actual value is equal to the given value 052 */ 053 EQUAL("eq"), 054 055 /** 056 * Code Value: <b>gt</b> 057 * 058 * The actual value is greater than the given value. 059 */ 060 GREATERTHAN("gt"), 061 062 /** 063 * Code Value: <b>ge</b> 064 * 065 * The actual value is greater than or equal to the given value. 066 */ 067 GREATERTHAN_OR_EQUALS("ge"), 068 069 /** 070 * Code Value: <b>lt</b> 071 * 072 * The actual value is less than the given value. 073 */ 074 LESSTHAN("lt"), 075 076 /** 077 * Code Value: <b>le</b> 078 * 079 * The actual value is less than or equal to the given value. 080 */ 081 LESSTHAN_OR_EQUALS("le"), 082 083 /** 084 * Code Value: <b>ne</b> 085 * 086 * The actual value is not equal to the given value 087 */ 088 NOT_EQUAL("ne"), 089 090 /** 091 * Code Value: <b>sa</b> 092 * 093 * The range of the search value does not overlap with the range of the target value, and the range below the search value contains the range of the target value 094 */ 095 STARTS_AFTER("sa"); 096 097 private static final Map<String, ParamPrefixEnum> VALUE_TO_PREFIX; 098 099 static { 100 HashMap<String, ParamPrefixEnum> valueToPrefix = new HashMap<String, ParamPrefixEnum>(); 101 for (ParamPrefixEnum next : values()) { 102 valueToPrefix.put(next.getValue(), next); 103 } 104 105 VALUE_TO_PREFIX = Collections.unmodifiableMap(valueToPrefix); 106 } 107 108 private final String myValue; 109 110 private ParamPrefixEnum(String theValue) { 111 myValue = theValue; 112 } 113 114 /** 115 * Returns the value, e.g. <code>lt</code> or <code>eq</code> 116 */ 117 public String getValue() { 118 return myValue; 119 } 120 121 /** 122 * Returns the prefix associated with a given DSTU2+ value (e.g. <code>lt</code> or <code>eq</code>) 123 * 124 * @param theValue 125 * e.g. <code><</code> or <code>~</code> 126 * @return The prefix, or <code>null</code> if no prefix matches the value 127 */ 128 public static ParamPrefixEnum forValue(String theValue) { 129 return VALUE_TO_PREFIX.get(theValue); 130 } 131}