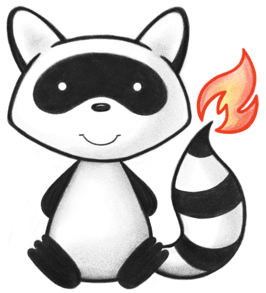
001/*- 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.param; 021 022import java.util.Set; 023 024public class QualifierDetails { 025 026 private String myColonQualifier; 027 private String myDotQualifier; 028 private String myParamName; 029 private String myWholeQualifier; 030 031 public boolean passes(Set<String> theQualifierWhitelist, Set<String> theQualifierBlacklist) { 032 if (theQualifierWhitelist != null) { 033 if (!theQualifierWhitelist.contains(".*")) { 034 if (myDotQualifier != null) { 035 if (!theQualifierWhitelist.contains(myDotQualifier)) { 036 return false; 037 } 038 } else { 039 if (!theQualifierWhitelist.contains(".")) { 040 return false; 041 } 042 } 043 } 044 } 045 if (theQualifierBlacklist != null) { 046 if (myDotQualifier != null) { 047 if (theQualifierBlacklist.contains(myDotQualifier)) { 048 return false; 049 } 050 } 051 if (myColonQualifier != null) { 052 if (theQualifierBlacklist.contains(myColonQualifier)) { 053 return false; 054 } 055 } 056 } 057 058 return true; 059 } 060 061 public void setParamName(String theParamName) { 062 myParamName = theParamName; 063 } 064 065 public String getParamName() { 066 return myParamName; 067 } 068 069 public void setColonQualifier(String theColonQualifier) { 070 myColonQualifier = theColonQualifier; 071 } 072 073 public void setDotQualifier(String theDotQualifier) { 074 myDotQualifier = theDotQualifier; 075 } 076 077 public String getWholeQualifier() { 078 return myWholeQualifier; 079 } 080 081 public void setWholeQualifier(String theWholeQualifier) { 082 myWholeQualifier = theWholeQualifier; 083 } 084 085 public static QualifierDetails extractQualifiersFromParameterName(String theParamName) { 086 QualifierDetails retVal = new QualifierDetails(); 087 if (theParamName == null || theParamName.length() == 0) { 088 return retVal; 089 } 090 091 int dotIdx = -1; 092 int colonIdx = -1; 093 for (int idx = 0; idx < theParamName.length(); idx++) { 094 char nextChar = theParamName.charAt(idx); 095 if (nextChar == '.' && dotIdx == -1) { 096 dotIdx = idx; 097 } else if (nextChar == ':' && colonIdx == -1) { 098 colonIdx = idx; 099 } 100 } 101 102 if (dotIdx != -1 && colonIdx != -1) { 103 if (dotIdx < colonIdx) { 104 retVal.setDotQualifier(theParamName.substring(dotIdx, colonIdx)); 105 retVal.setColonQualifier(theParamName.substring(colonIdx)); 106 retVal.setParamName(theParamName.substring(0, dotIdx)); 107 retVal.setWholeQualifier(theParamName.substring(dotIdx)); 108 } else { 109 retVal.setColonQualifier(theParamName.substring(colonIdx, dotIdx)); 110 retVal.setDotQualifier(theParamName.substring(dotIdx)); 111 retVal.setParamName(theParamName.substring(0, colonIdx)); 112 retVal.setWholeQualifier(theParamName.substring(colonIdx)); 113 } 114 } else if (dotIdx != -1) { 115 retVal.setDotQualifier(theParamName.substring(dotIdx)); 116 retVal.setParamName(theParamName.substring(0, dotIdx)); 117 retVal.setWholeQualifier(theParamName.substring(dotIdx)); 118 } else if (colonIdx != -1) { 119 retVal.setColonQualifier(theParamName.substring(colonIdx)); 120 retVal.setParamName(theParamName.substring(0, colonIdx)); 121 retVal.setWholeQualifier(theParamName.substring(colonIdx)); 122 } else { 123 retVal.setParamName(theParamName); 124 retVal.setColonQualifier(null); 125 retVal.setDotQualifier(null); 126 retVal.setWholeQualifier(null); 127 } 128 129 return retVal; 130 } 131}