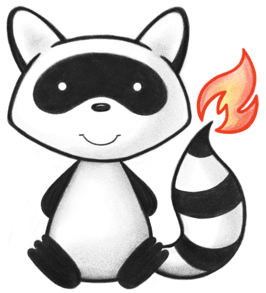
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.param; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.model.primitive.UriDt; 024import ca.uhn.fhir.rest.api.Constants; 025import org.apache.commons.lang3.StringUtils; 026import org.apache.commons.lang3.builder.EqualsBuilder; 027import org.apache.commons.lang3.builder.HashCodeBuilder; 028import org.apache.commons.lang3.builder.ToStringBuilder; 029import org.apache.commons.lang3.builder.ToStringStyle; 030import org.slf4j.Logger; 031import org.slf4j.LoggerFactory; 032 033import static org.apache.commons.lang3.StringUtils.defaultString; 034 035public class SpecialParam extends BaseParam /*implements IQueryParameterType*/ { 036 private static final Logger ourLog = LoggerFactory.getLogger(StringParam.class); 037 038 private String myValue; 039 private boolean myContains; 040 041 /** 042 * Constructor 043 */ 044 public SpecialParam() { 045 super(); 046 } 047 048 @Override 049 String doGetQueryParameterQualifier() { 050 if (myContains) { 051 return Constants.PARAMQUALIFIER_STRING_CONTAINS; 052 } else { 053 return null; 054 } 055 } 056 057 /** 058 * {@inheritDoc} 059 */ 060 @Override 061 String doGetValueAsQueryToken(FhirContext theContext) { 062 return ParameterUtil.escape(getValue()); 063 } 064 065 /** 066 * {@inheritDoc} 067 */ 068 @Override 069 void doSetValueAsQueryToken(FhirContext theContext, String theParamName, String theQualifier, String theParameter) { 070 if (Constants.PARAMQUALIFIER_STRING_CONTAINS.equals(theQualifier)) { 071 if (theParamName.equalsIgnoreCase(Constants.PARAM_TEXT) 072 || theParamName.equalsIgnoreCase(Constants.PARAM_CONTENT)) { 073 setContains(true); 074 } else { 075 ourLog.debug( 076 "Attempted to set the :contains modifier on a special search parameter that was not `_text` or `_content`. This is not supported."); 077 } 078 } 079 setValue(ParameterUtil.unescape(theParameter)); 080 } 081 082 /** 083 * Returns the value for the token (generally the value to the right of the 084 * vertical bar on the URL) 085 */ 086 public String getValue() { 087 return myValue; 088 } 089 090 public String getValueNotNull() { 091 return defaultString(myValue); 092 } 093 094 public boolean isEmpty() { 095 return StringUtils.isEmpty(myValue); 096 } 097 098 public SpecialParam setValue(String theValue) { 099 myValue = theValue; 100 return this; 101 } 102 103 @Override 104 public String toString() { 105 ToStringBuilder builder = new ToStringBuilder(this, ToStringStyle.SHORT_PREFIX_STYLE); 106 builder.append("value", getValue()); 107 if (getMissing() != null) { 108 builder.append(":missing", getMissing()); 109 } 110 return builder.toString(); 111 } 112 113 private static String toSystemValue(UriDt theSystem) { 114 return theSystem.getValueAsString(); 115 } 116 /** 117 * Special parameter modifier <code>:contains</code> for _text and _content 118 */ 119 public boolean isContains() { 120 return myContains; 121 } 122 123 /** 124 * Special parameter modifier <code>:contains</code> for _text and _content 125 */ 126 public SpecialParam setContains(boolean theContains) { 127 myContains = theContains; 128 if (myContains) { 129 setMissing(null); 130 } 131 return this; 132 } 133 134 @Override 135 public int hashCode() { 136 return new HashCodeBuilder(17, 37) 137 .append(isContains()) 138 .append(getValue()) 139 .append(getMissing()) 140 .toHashCode(); 141 } 142 143 @Override 144 public boolean equals(Object obj) { 145 if (this == obj) { 146 return true; 147 } 148 if (obj == null) { 149 return false; 150 } 151 if (!(obj instanceof SpecialParam)) { 152 return false; 153 } 154 155 SpecialParam other = (SpecialParam) obj; 156 157 EqualsBuilder eb = new EqualsBuilder(); 158 eb.append(myContains, other.myContains); 159 eb.append(myValue, other.myValue); 160 eb.append(getMissing(), other.getMissing()); 161 162 return eb.isEquals(); 163 } 164}