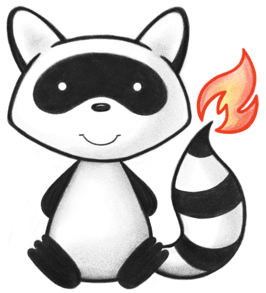
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.param; 021 022import ca.uhn.fhir.model.base.composite.BaseCodingDt; 023import ca.uhn.fhir.model.base.composite.BaseIdentifierDt; 024import ca.uhn.fhir.util.CoverageIgnore; 025 026import java.util.ArrayList; 027import java.util.List; 028 029/** 030 * This class represents a restful search operation parameter for an "OR list" of tokens (in other words, a 031 * list which can contain one-or-more tokens, where the server should return results matching any of the tokens) 032 */ 033public class TokenOrListParam extends BaseOrListParam<TokenOrListParam, TokenParam> { 034 035 /** 036 * Create a new empty token "OR list" 037 */ 038 public TokenOrListParam() {} 039 040 /** 041 * Create a new token "OR list" with a single token, or multiple tokens which have the same system value 042 * 043 * @param theSystem 044 * The system to use for the one token to pre-populate in this list 045 * @param theValues 046 * The values to use for the one token to pre-populate in this list 047 */ 048 public TokenOrListParam(String theSystem, String... theValues) { 049 for (String next : theValues) { 050 add(theSystem, next); 051 } 052 } 053 054 /** 055 * Convenience method which adds a token to this OR list using the system and code from a coding 056 */ 057 public void add(BaseCodingDt theCodingDt) { 058 add(new TokenParam(theCodingDt)); 059 } 060 061 /** 062 * Convenience method which adds a token to this OR list using the system and value from an identifier 063 */ 064 public void add(BaseIdentifierDt theIdentifierDt) { 065 add(new TokenParam(theIdentifierDt)); 066 } 067 068 /** 069 * Add a new token to this list 070 * @param theSystem 071 * The system to use for the one token to pre-populate in this list 072 */ 073 public TokenOrListParam add(String theSystem, String theValue) { 074 add(new TokenParam(theSystem, theValue)); 075 return this; 076 } 077 078 /** 079 * Add a new token to this list 080 */ 081 public TokenOrListParam add(String theValue) { 082 add(new TokenParam(null, theValue)); 083 return this; 084 } 085 086 public List<BaseCodingDt> getListAsCodings() { 087 ArrayList<BaseCodingDt> retVal = new ArrayList<BaseCodingDt>(); 088 for (TokenParam next : getValuesAsQueryTokens()) { 089 InternalCodingDt nextCoding = next.getValueAsCoding(); 090 if (!nextCoding.isEmpty()) { 091 retVal.add(nextCoding); 092 } 093 } 094 return retVal; 095 } 096 097 @CoverageIgnore 098 @Override 099 TokenParam newInstance() { 100 return new TokenParam(); 101 } 102 103 public boolean doesCodingListMatch(List<? extends BaseCodingDt> theCodings) { 104 List<BaseCodingDt> paramCodings = getListAsCodings(); 105 for (BaseCodingDt coding : theCodings) { 106 for (BaseCodingDt paramCoding : paramCodings) { 107 if (coding.matchesToken(paramCoding)) { 108 return true; 109 } 110 } 111 } 112 return false; 113 } 114 115 @CoverageIgnore 116 @Override 117 public TokenOrListParam addOr(TokenParam theParameter) { 118 add(theParameter); 119 return this; 120 } 121}