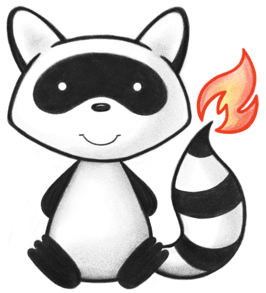
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.param; 021 022import ca.uhn.fhir.rest.api.Constants; 023 024import java.util.HashMap; 025import java.util.Map; 026 027/** 028 * Modifiers for {@link TokenParam} 029 */ 030public enum TokenParamModifier { 031 /** 032 * :above 033 */ 034 ABOVE(":above"), 035 036 /** 037 * :above 038 */ 039 BELOW(":below"), 040 041 /** 042 * :in 043 */ 044 IN(":in"), 045 046 /** 047 * :not 048 */ 049 NOT(":not"), 050 051 /** 052 * :not-in 053 */ 054 NOT_IN(":not-in"), 055 056 /** 057 * :text 058 */ 059 TEXT(Constants.PARAMQUALIFIER_TOKEN_TEXT), 060 061 /** 062 * :of-type 063 */ 064 OF_TYPE(Constants.PARAMQUALIFIER_TOKEN_OF_TYPE); 065 066 private static final Map<String, TokenParamModifier> VALUE_TO_ENUM; 067 068 static { 069 Map<String, TokenParamModifier> valueToEnum = new HashMap<String, TokenParamModifier>(); 070 for (TokenParamModifier next : values()) { 071 valueToEnum.put(next.getValue(), next); 072 } 073 VALUE_TO_ENUM = valueToEnum; 074 } 075 076 private final String myValue; 077 078 private TokenParamModifier(String theValue) { 079 myValue = theValue; 080 } 081 082 public String getValue() { 083 return myValue; 084 } 085 086 /** 087 * The modifier without the : 088 * @return the string after the leading : 089 */ 090 public String getBareModifier() { 091 return myValue.substring(1); 092 } 093 094 public static TokenParamModifier forValue(String theValue) { 095 return VALUE_TO_ENUM.get(theValue); 096 } 097 098 public boolean isNegative() { 099 switch (this) { 100 case NOT: 101 case NOT_IN: 102 return true; 103 default: 104 return false; 105 } 106 } 107}