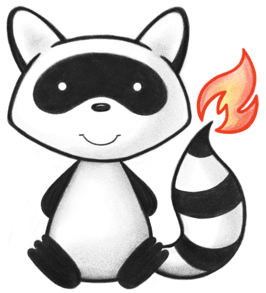
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.param; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.model.api.IQueryParameterType; 024import ca.uhn.fhir.model.primitive.StringDt; 025import ca.uhn.fhir.model.primitive.UriDt; 026import org.apache.commons.lang3.StringUtils; 027import org.apache.commons.lang3.builder.ToStringBuilder; 028import org.apache.commons.lang3.builder.ToStringStyle; 029 030import static org.apache.commons.lang3.StringUtils.defaultString; 031 032public class UriParam extends BaseParam implements IQueryParameterType { 033 034 private UriParamQualifierEnum myQualifier; 035 private String myValue; 036 037 /** 038 * Constructor 039 */ 040 public UriParam() { 041 super(); 042 } 043 044 public UriParam(String theValue) { 045 setValue(theValue); 046 } 047 048 @Override 049 String doGetQueryParameterQualifier() { 050 return myQualifier != null ? myQualifier.getValue() : null; 051 } 052 053 @Override 054 String doGetValueAsQueryToken(FhirContext theContext) { 055 return ParameterUtil.escape(myValue); 056 } 057 058 @Override 059 void doSetValueAsQueryToken(FhirContext theContext, String theParamName, String theQualifier, String theValue) { 060 myQualifier = UriParamQualifierEnum.forValue(theQualifier); 061 myValue = ParameterUtil.unescape(theValue); 062 } 063 064 /** 065 * Gets the qualifier for this param (may be <code>null</code> and generally will be) 066 */ 067 public UriParamQualifierEnum getQualifier() { 068 return myQualifier; 069 } 070 071 public String getValue() { 072 return myValue; 073 } 074 075 public StringDt getValueAsStringDt() { 076 return new StringDt(myValue); 077 } 078 079 public UriDt getValueAsUriDt() { 080 return new UriDt(myValue); 081 } 082 083 public String getValueNotNull() { 084 return defaultString(myValue); 085 } 086 087 public boolean isEmpty() { 088 return StringUtils.isEmpty(myValue); 089 } 090 091 /** 092 * Sets the qualifier for this param (may be <code>null</code> and generally will be) 093 * 094 * @return Returns a reference to <code>this</code> for easy method chanining 095 */ 096 public UriParam setQualifier(UriParamQualifierEnum theQualifier) { 097 myQualifier = theQualifier; 098 return this; 099 } 100 101 /** 102 * Sets the value for this param 103 * 104 * @return Returns a reference to <code>this</code> for easy method chanining 105 */ 106 public UriParam setValue(String theValue) { 107 myValue = theValue; 108 return this; 109 } 110 111 @Override 112 public String toString() { 113 ToStringBuilder builder = new ToStringBuilder(this, ToStringStyle.SHORT_PREFIX_STYLE); 114 builder.append("value", getValue()); 115 return builder.toString(); 116 } 117}