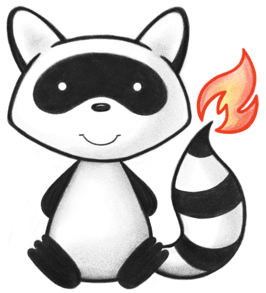
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.param; 021 022import java.util.Collections; 023import java.util.HashMap; 024import java.util.Map; 025 026/** 027 * Qualifiers for {@link UriParam} 028 */ 029public enum UriParamQualifierEnum { 030 031 /** 032 * The search parameter is a concept with the form <code>[system]|[code]</code>, 033 * and the search parameter tests whether the coding in a resource subsumes the 034 * specified search code. For example, the search concept has an is-a relationship 035 * with the coding in the resource, and this includes the coding itself. 036 * <p> 037 * Value <code>:above</code> 038 * </p> 039 */ 040 ABOVE(":above"), 041 042 /** 043 * The search parameter is a concept with the form <code>[system]|[code]</code>, 044 * and the search parameter tests whether the coding in a resource subsumes the 045 * specified search code. For example, the search concept has an is-a relationship 046 * with the coding in the resource, and this includes the coding itself. 047 * <p> 048 * Value <code>:below</code> 049 * </p> 050 */ 051 BELOW(":below"), 052 053 /** 054 * The contains modifier allows clients to indicate that a supplied URI input should be matched 055 * as a case-insensitive and combining-character insensitive match anywhere in the target URI. 056 * <p> 057 * Value <code>:contains</code> 058 * </p> 059 */ 060 CONTAINS(":contains"); 061 062 private static final Map<String, UriParamQualifierEnum> KEY_TO_VALUE; 063 064 static { 065 HashMap<String, UriParamQualifierEnum> key2value = new HashMap<String, UriParamQualifierEnum>(); 066 for (UriParamQualifierEnum next : values()) { 067 key2value.put(next.getValue(), next); 068 } 069 KEY_TO_VALUE = Collections.unmodifiableMap(key2value); 070 } 071 072 private final String myValue; 073 074 private UriParamQualifierEnum(String theValue) { 075 myValue = theValue; 076 } 077 078 /** 079 * Returns the qualifier value, e.g. <code>:below</code> 080 */ 081 public String getValue() { 082 return myValue; 083 } 084 085 /** 086 * Returns the {@link UriParamQualifierEnum} matching the given qualifier value, such as <code>:below</code>, 087 * or <code>null</code> 088 */ 089 public static UriParamQualifierEnum forValue(String theValue) { 090 return KEY_TO_VALUE.get(theValue); 091 } 092}