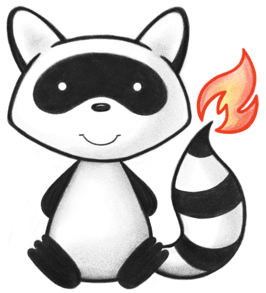
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.param.binder; 021 022import ca.uhn.fhir.context.ConfigurationException; 023import ca.uhn.fhir.i18n.Msg; 024 025import java.util.ArrayList; 026import java.util.Collection; 027import java.util.HashSet; 028import java.util.List; 029import java.util.Set; 030 031public class CollectionBinder 032// implements IParamBinder 033{ 034 035 /** 036 * @param thePositionDescription Just used in exceptions if theCollectionType is invalid 037 */ 038 @SuppressWarnings({"rawtypes", "cast"}) 039 public static Class<? extends Collection> getInstantiableCollectionType( 040 Class<? extends Collection<?>> theCollectionType, String thePositionDescription) { 041 if (theCollectionType.equals(List.class) || theCollectionType.equals(ArrayList.class)) { 042 return (Class<? extends Collection>) ArrayList.class; 043 } else if (theCollectionType.equals(Set.class) || theCollectionType.equals(HashSet.class)) { 044 return (Class<? extends Collection>) HashSet.class; 045 } else if (theCollectionType.equals(Collection.class)) { 046 return (Class<? extends Collection>) ArrayList.class; 047 } else { 048 throw new ConfigurationException(Msg.code(1956) + "Unsupported binding collection type '" 049 + theCollectionType.getCanonicalName() + "' for " + thePositionDescription); 050 } 051 } 052 053 // private Class<?> myCollectionType; 054 // private IParamBinder myWrap; 055 // 056 // public CollectionBinder(IParamBinder theWrap, Class<? extends java.util.Collection<?>> theCollectionType) { 057 // myWrap = theWrap; 058 // if (theCollectionType == List.class || theCollectionType == ArrayList.class) { 059 // myCollectionType = ArrayList.class; 060 // } else if (theCollectionType == Set.class || theCollectionType == HashSet.class) { 061 // myCollectionType = HashSet.class; 062 // } else if (theCollectionType == Collection.class) { 063 // myCollectionType = ArrayList.class; 064 // } else { 065 // throw new ConfigurationException(Msg.code(1957) + "Unsupported binding collection type: " + 066 // theCollectionType.getCanonicalName()); 067 // } 068 // } 069 070 // @Override 071 // public String encode(Object theString) throws InternalErrorException { 072 // Collection<?> obj = (Collection<?>) theString; 073 // StringBuilder b = new StringBuilder(); 074 // for (Object object : obj) { 075 // String next = myWrap.encode(object); 076 // if (b.length() > 0) { 077 // b.append(","); 078 // } 079 // b.append(next.replace(",", "\\,")); 080 // } 081 // return b.toString(); 082 // } 083 // 084 // @SuppressWarnings("unchecked") 085 // @Override 086 // public Object parse(String theString) throws InternalErrorException { 087 // Collection<Object> retVal; 088 // try { 089 // retVal = (Collection<Object>) myCollectionType.newInstance(); 090 // } catch (Exception e) { 091 // throw new InternalErrorException(Msg.code(1958) + "Failed to instantiate " + myCollectionType, e); 092 // } 093 // 094 // List<String> params = QueryUtil.splitQueryStringByCommasIgnoreEscape(theString); 095 // for (String string : params) { 096 // Object nextParsed = myWrap.parse(string); 097 // retVal.add(nextParsed); 098 // } 099 // 100 // return retVal; 101 // } 102 103}