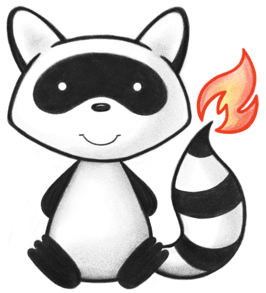
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.param.binder; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.i18n.Msg; 024import ca.uhn.fhir.model.api.IQueryParameterOr; 025import ca.uhn.fhir.model.api.IQueryParameterType; 026import ca.uhn.fhir.rest.api.QualifiedParamList; 027import ca.uhn.fhir.rest.server.exceptions.InternalErrorException; 028import ca.uhn.fhir.rest.server.exceptions.InvalidRequestException; 029 030import java.util.Collections; 031import java.util.List; 032 033public final class QueryParameterOrBinder extends BaseBinder<IQueryParameterOr<?>> 034 implements IParamBinder<IQueryParameterOr<?>> { 035 036 public QueryParameterOrBinder( 037 Class<? extends IQueryParameterOr<?>> theType, 038 List<Class<? extends IQueryParameterType>> theCompositeTypes) { 039 super(theType, theCompositeTypes); 040 } 041 042 @SuppressWarnings("unchecked") 043 @Override 044 public List<IQueryParameterOr<?>> encode(FhirContext theContext, IQueryParameterOr<?> theValue) 045 throws InternalErrorException { 046 IQueryParameterOr<?> retVal = (theValue); 047 List<?> retVal2 = Collections.singletonList((IQueryParameterOr<?>) retVal); 048 return (List<IQueryParameterOr<?>>) retVal2; 049 } 050 051 @Override 052 public IQueryParameterOr<?> parse(FhirContext theContext, String theParamName, List<QualifiedParamList> theString) 053 throws InternalErrorException, InvalidRequestException { 054 IQueryParameterOr<?> dt; 055 try { 056 dt = newInstance(); 057 if (theString.size() == 0 || theString.get(0).size() == 0) { 058 return dt; 059 } 060 if (theString.size() > 1) { 061 throw new InvalidRequestException( 062 Msg.code(1953) + "Multiple values detected for non-repeatable parameter '" + theParamName 063 + "'. This server is not configured to allow multiple (AND/OR) values for this param."); 064 } 065 066 dt.setValuesAsQueryTokens(theContext, theParamName, theString.get(0)); 067 } catch (SecurityException e) { 068 throw new InternalErrorException(Msg.code(1954) + e); 069 } 070 return dt; 071 } 072}