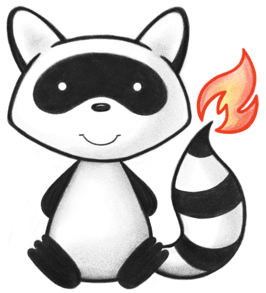
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.server.exceptions; 021 022import ca.uhn.fhir.rest.api.Constants; 023import ca.uhn.fhir.util.CoverageIgnore; 024 025/** 026 * Represents an <b>HTTP 401 Client Unauthorized</b> response, which 027 * means that the client needs to provide credentials, or has 028 * provided invalid credentials. 029 * <p> 030 * For security failures, you should use 031 * {@link AuthenticationException} if you want to indicate that the 032 * user could not be authenticated (e.g. credential failures), also 033 * known as an <b>authentication</b> failure. 034 * You should use {@link ForbiddenOperationException} if you want to 035 * indicate that the authenticated user does not have permission to 036 * perform the requested operation, also known as an <b>authorization</b> 037 * failure. 038 * </p> 039 * <p> 040 * Note that a complete list of RESTful exceptions is available in the <a href="./package-summary.html">Package 041 * Summary</a>. 042 * </p> 043 * 044 */ 045@CoverageIgnore 046public class AuthenticationException extends BaseServerResponseException { 047 048 public static final int STATUS_CODE = Constants.STATUS_HTTP_401_CLIENT_UNAUTHORIZED; 049 050 private static final long serialVersionUID = 1L; 051 052 public AuthenticationException() { 053 super(STATUS_CODE, "Client unauthorized"); 054 } 055 056 public AuthenticationException(String theMessage) { 057 super(STATUS_CODE, theMessage); 058 } 059 060 public AuthenticationException(String theMessage, Throwable theCause) { 061 super(STATUS_CODE, theMessage, theCause); 062 } 063 064 /** 065 * Adds a <code>WWW-Authenticate</code> header to the response, of the form:<br/> 066 * <code>WWW-Authenticate: Basic realm="theRealm"</code> 067 * 068 * @return Returns a reference to <code>this</code> for easy method chaining 069 */ 070 public AuthenticationException addAuthenticateHeaderForRealm(String theRealm) { 071 addResponseHeader("WWW-Authenticate", "Basic realm=\"" + theRealm + "\""); 072 return this; 073 } 074}