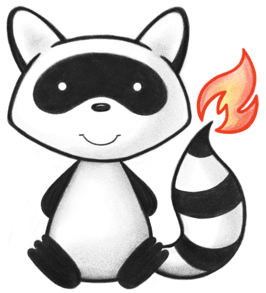
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.server.exceptions; 021 022import ca.uhn.fhir.rest.api.Constants; 023import ca.uhn.fhir.util.CoverageIgnore; 024import org.hl7.fhir.instance.model.api.IBaseOperationOutcome; 025 026/** 027 * This Represents an <b>HTTP 403 Forbidden</b> response, which generally indicates one of two conditions: 028 * <ul> 029 * <li>Authentication was provided, but the authenticated user is not permitted to perform the requested operation.</li> 030 * <li>The operation is forbidden to all users. Repeating the request with authentication would serve no purpose.</li> 031 * </ul> 032 * 033 * <p> 034 * For security failures, you should use 035 * {@link AuthenticationException} if you want to indicate that the 036 * user could not be authenticated (e.g. credential failures), also 037 * known as an <b>authentication</b> failure. 038 * You should use {@link ForbiddenOperationException} if you want to 039 * indicate that the authenticated user does not have permission to 040 * perform the requested operation, also known as an <b>authorization</b> 041 * failure. 042 * </p> 043 * <p> 044 * Note that a complete list of RESTful exceptions is available in the <a href="./package-summary.html">Package 045 * Summary</a>. 046 * </p> 047 */ 048@CoverageIgnore 049public class ForbiddenOperationException extends BaseServerResponseException { 050 051 public static final int STATUS_CODE = Constants.STATUS_HTTP_403_FORBIDDEN; 052 private static final long serialVersionUID = 1L; 053 054 public ForbiddenOperationException(String theMessage) { 055 super(STATUS_CODE, theMessage); 056 } 057 058 /** 059 * Constructor 060 * 061 * @param theMessage 062 * The message 063 * @param theOperationOutcome 064 * The OperationOutcome resource to return to the client 065 */ 066 public ForbiddenOperationException(String theMessage, IBaseOperationOutcome theOperationOutcome) { 067 super(STATUS_CODE, theMessage, theOperationOutcome); 068 } 069}