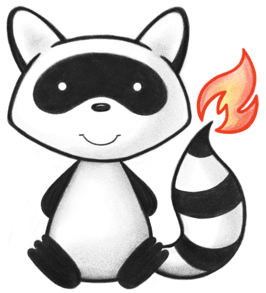
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.server.exceptions; 021 022import ca.uhn.fhir.rest.api.Constants; 023import ca.uhn.fhir.util.CoverageIgnore; 024import org.hl7.fhir.instance.model.api.IBaseOperationOutcome; 025 026/** 027 * Represents an <b>HTTP 500 Internal Error</b> response. 028 * This status indicates that the server failed to successfully process the 029 * request. This generally means that the server is misbehaving or is 030 * misconfigured in some way, although a misbehaving server might also 031 * send this status code in the case of a bad request message (although it 032 * should not do this; an HTTP 4xx response is more appropriate in that 033 * situation). 034 * 035 * <p> 036 * Note that a complete list of RESTful exceptions is available in the 037 * <a href="./package-summary.html">Package Summary</a>. 038 * </p> 039 * 040 * @see UnprocessableEntityException Which should be used for business level validation failures 041 */ 042@CoverageIgnore 043public class InternalErrorException extends BaseServerResponseException { 044 045 public static final int STATUS_CODE = Constants.STATUS_HTTP_500_INTERNAL_ERROR; 046 047 private static final long serialVersionUID = 1L; 048 049 /** 050 * Constructor 051 * 052 * @param theMessage 053 * The message 054 * @param theOperationOutcome The OperationOutcome resource to return to the client 055 */ 056 public InternalErrorException(String theMessage, IBaseOperationOutcome theOperationOutcome) { 057 super(STATUS_CODE, theMessage, theOperationOutcome); 058 } 059 060 public InternalErrorException(String theMessage) { 061 super(STATUS_CODE, theMessage); 062 } 063 064 public InternalErrorException(String theMessage, Throwable theCause) { 065 super(STATUS_CODE, theMessage, theCause); 066 } 067 068 public InternalErrorException(Throwable theCause) { 069 super(STATUS_CODE, theCause); 070 } 071}