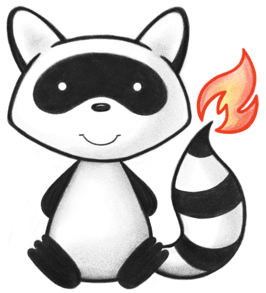
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.server.exceptions; 021 022import ca.uhn.fhir.rest.api.Constants; 023import ca.uhn.fhir.util.CoverageIgnore; 024import org.hl7.fhir.instance.model.api.IBaseOperationOutcome; 025 026/** 027 * Represents an <b>HTTP 400 Bad Request</b> response. 028 * This status indicates that the client's message was invalid (e.g. not a valid FHIR Resource 029 * per the specifications), as opposed to the {@link UnprocessableEntityException} which indicates 030 * that data does not pass business rule validation on the server. 031 * 032 * <p> 033 * Note that a complete list of RESTful exceptions is available in the 034 * <a href="./package-summary.html">Package Summary</a>. 035 * </p> 036 * 037 * @see UnprocessableEntityException Which should be used for business level validation failures 038 */ 039@CoverageIgnore 040public class InvalidRequestException extends BaseServerResponseException { 041 042 public static final int STATUS_CODE = Constants.STATUS_HTTP_400_BAD_REQUEST; 043 private static final long serialVersionUID = 1L; 044 045 /** 046 * Constructor 047 */ 048 public InvalidRequestException(String theMessage) { 049 super(STATUS_CODE, theMessage); 050 } 051 052 /** 053 * Constructor 054 */ 055 public InvalidRequestException(String theMessage, Throwable theCause) { 056 super(STATUS_CODE, theMessage, theCause); 057 } 058 059 /** 060 * Constructor 061 */ 062 public InvalidRequestException(Throwable theCause) { 063 super(STATUS_CODE, theCause); 064 } 065 066 /** 067 * Constructor 068 * 069 * @param theMessage 070 * The message 071 * @param theOperationOutcome The OperationOutcome resource to return to the client 072 */ 073 public InvalidRequestException(String theMessage, IBaseOperationOutcome theOperationOutcome) { 074 super(STATUS_CODE, theMessage, theOperationOutcome); 075 } 076}