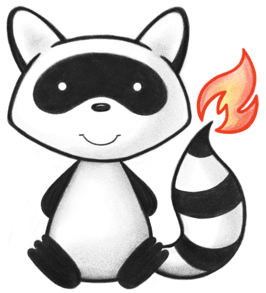
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.server.exceptions; 021 022import ca.uhn.fhir.model.base.composite.BaseIdentifierDt; 023import ca.uhn.fhir.rest.api.Constants; 024import ca.uhn.fhir.util.CoverageIgnore; 025import org.hl7.fhir.instance.model.api.IBaseOperationOutcome; 026import org.hl7.fhir.instance.model.api.IBaseResource; 027import org.hl7.fhir.instance.model.api.IIdType; 028 029/** 030 * Represents an <b>HTTP 410 Resource Gone</b> response, which geenerally 031 * indicates that the resource has been deleted 032 */ 033@CoverageIgnore 034public class ResourceGoneException extends BaseServerResponseException { 035 036 public static final int STATUS_CODE = Constants.STATUS_HTTP_410_GONE; 037 private static final long serialVersionUID = 1L; 038 private IIdType myResourceId; 039 040 /** 041 * Constructor which creates an error message based on a given resource ID 042 * 043 * @param theResourceId The ID of the resource that could not be found 044 */ 045 public ResourceGoneException(IIdType theResourceId) { 046 super(STATUS_CODE, "Resource " + (theResourceId != null ? theResourceId.getValue() : "") + " is gone/deleted"); 047 myResourceId = theResourceId; 048 } 049 050 /** 051 * @deprecated This constructor has a dependency on a specific model version and will be removed. Deprecated in HAPI 052 * 1.6 - 2016-07-02 053 */ 054 @Deprecated 055 public ResourceGoneException(Class<? extends IBaseResource> theClass, BaseIdentifierDt thePatientId) { 056 super( 057 STATUS_CODE, 058 "Resource of type " + theClass.getSimpleName() + " with ID " + thePatientId + " is gone/deleted"); 059 myResourceId = null; 060 } 061 062 /** 063 * Constructor which creates an error message based on a given resource ID 064 * 065 * @param theClass The type of resource that could not be found 066 * @param theResourceId The ID of the resource that could not be found 067 */ 068 public ResourceGoneException(Class<? extends IBaseResource> theClass, IIdType theResourceId) { 069 super( 070 STATUS_CODE, 071 "Resource of type " + theClass.getSimpleName() + " with ID " + theResourceId + " is gone/deleted"); 072 myResourceId = theResourceId; 073 } 074 075 /** 076 * Constructor 077 * 078 * @param theMessage The message 079 * @param theOperationOutcome The OperationOutcome resource to return to the client 080 */ 081 public ResourceGoneException(String theMessage, IBaseOperationOutcome theOperationOutcome) { 082 super(STATUS_CODE, theMessage, theOperationOutcome); 083 } 084 085 /** 086 * Constructor 087 * 088 * @param theMessage The message 089 */ 090 public ResourceGoneException(String theMessage) { 091 super(STATUS_CODE, theMessage); 092 } 093 094 public IIdType getResourceId() { 095 return myResourceId; 096 } 097 098 public void setResourceId(IIdType theResourceId) { 099 myResourceId = theResourceId; 100 } 101}