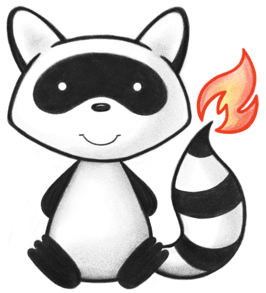
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.server.exceptions; 021 022import ca.uhn.fhir.i18n.Msg; 023import ca.uhn.fhir.model.api.IResource; 024import ca.uhn.fhir.model.base.composite.BaseIdentifierDt; 025import ca.uhn.fhir.model.primitive.IdDt; 026import ca.uhn.fhir.rest.api.Constants; 027import ca.uhn.fhir.util.CoverageIgnore; 028import org.hl7.fhir.instance.model.api.IBaseOperationOutcome; 029import org.hl7.fhir.instance.model.api.IIdType; 030 031/** 032 * Represents an <b>HTTP 404 Resource Not Found</b> response, which means that the request is pointing to a resource that does not exist. 033 */ 034@CoverageIgnore 035public class ResourceNotFoundException extends BaseServerResponseException { 036 037 private static final long serialVersionUID = 1L; 038 039 public static final int STATUS_CODE = Constants.STATUS_HTTP_404_NOT_FOUND; 040 041 public ResourceNotFoundException(Class<? extends IResource> theClass, IdDt theId) { 042 super(STATUS_CODE, createErrorMessage(theClass, theId)); 043 } 044 045 public ResourceNotFoundException( 046 Class<? extends IResource> theClass, IdDt theId, IBaseOperationOutcome theOperationOutcome) { 047 super(STATUS_CODE, createErrorMessage(theClass, theId), theOperationOutcome); 048 } 049 050 public ResourceNotFoundException(Class<? extends IResource> theClass, IIdType theId) { 051 super(STATUS_CODE, createErrorMessage(theClass, theId)); 052 } 053 054 public ResourceNotFoundException( 055 Class<? extends IResource> theClass, IIdType theId, IBaseOperationOutcome theOperationOutcome) { 056 super(STATUS_CODE, createErrorMessage(theClass, theId), theOperationOutcome); 057 } 058 059 /** 060 * Constructor 061 * 062 * @param theMessage 063 * The message 064 * @param theOperationOutcome The OperationOutcome resource to return to the client 065 */ 066 public ResourceNotFoundException(String theMessage, IBaseOperationOutcome theOperationOutcome) { 067 super(STATUS_CODE, theMessage, theOperationOutcome); 068 } 069 070 /** 071 * @deprecated This doesn't make sense, since an identifier is not a resource ID and shouldn't generate a 404 if it isn't found - Should be removed 072 */ 073 @Deprecated 074 public ResourceNotFoundException(Class<? extends IResource> theClass, BaseIdentifierDt theId) { 075 super(STATUS_CODE, "Resource of type " + theClass.getSimpleName() + " with ID " + theId + " is not known"); 076 } 077 078 public ResourceNotFoundException(IdDt theId) { 079 super(STATUS_CODE, createErrorMessage(theId)); 080 } 081 082 public ResourceNotFoundException(IIdType theId) { 083 super(STATUS_CODE, createErrorMessage(theId)); 084 } 085 086 public ResourceNotFoundException(IIdType theId, IBaseOperationOutcome theOperationOutcome) { 087 super(STATUS_CODE, createErrorMessage(theId), theOperationOutcome); 088 } 089 090 public ResourceNotFoundException(String theMessage) { 091 super(STATUS_CODE, theMessage); 092 } 093 094 private static String createErrorMessage(Class<? extends IResource> theClass, IIdType theId) { 095 return Msg.code(970) + "Resource of type " + theClass.getSimpleName() + " with ID " + theId + " is not known"; 096 } 097 098 private static String createErrorMessage(IIdType theId) { 099 return Msg.code(971) + "Resource " + (theId != null ? theId.getValue() : "") + " is not known"; 100 } 101}