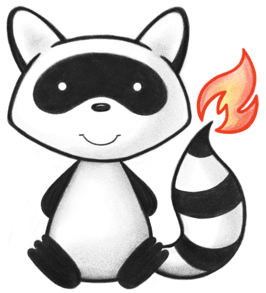
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.rest.server.exceptions; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.rest.api.Constants; 024import ca.uhn.fhir.util.CoverageIgnore; 025import ca.uhn.fhir.util.OperationOutcomeUtil; 026import org.hl7.fhir.instance.model.api.IBaseOperationOutcome; 027 028/** 029 * Represents an <b>HTTP 422 Unprocessable Entity</b> response, which means that a resource was rejected by the server because it "violated applicable FHIR profiles or server business rules". 030 * 031 * <p> 032 * This exception will generally contain an {@link IBaseOperationOutcome} instance which details the failure. 033 * </p> 034 * 035 * @see InvalidRequestException Which corresponds to an <b>HTTP 400 Bad Request</b> failure 036 */ 037@CoverageIgnore 038public class UnprocessableEntityException extends BaseServerResponseException { 039 040 public static final int STATUS_CODE = Constants.STATUS_HTTP_422_UNPROCESSABLE_ENTITY; 041 private static final String DEFAULT_MESSAGE = "Unprocessable Entity"; 042 private static final long serialVersionUID = 1L; 043 044 /** 045 * Constructor 046 * 047 * @param theMessage The message to add to the status line 048 * @param theOperationOutcome The {@link IBaseOperationOutcome} resource to return to the client 049 */ 050 public UnprocessableEntityException(String theMessage, IBaseOperationOutcome theOperationOutcome) { 051 super(STATUS_CODE, theMessage, theOperationOutcome); 052 } 053 054 /** 055 * Constructor which accepts an {@link IBaseOperationOutcome} resource which will be supplied in the response 056 * 057 * @deprecated Use constructor with FhirContext argument 058 */ 059 @Deprecated 060 public UnprocessableEntityException(IBaseOperationOutcome theOperationOutcome) { 061 super(STATUS_CODE, DEFAULT_MESSAGE, theOperationOutcome); 062 } 063 064 /** 065 * Constructor which accepts an {@link IBaseOperationOutcome} resource which will be supplied in the response 066 */ 067 public UnprocessableEntityException(FhirContext theCtx, IBaseOperationOutcome theOperationOutcome) { 068 super(STATUS_CODE, OperationOutcomeUtil.getFirstIssueDetails(theCtx, theOperationOutcome), theOperationOutcome); 069 } 070 071 /** 072 * Constructor which accepts a String describing the issue. This string will be translated into an {@link IBaseOperationOutcome} resource which will be supplied in the response. 073 */ 074 public UnprocessableEntityException(String theMessage) { 075 super(STATUS_CODE, theMessage); 076 } 077 078 /** 079 * Constructor which accepts a String describing the issue. This string will be translated into an {@link IBaseOperationOutcome} resource which will be supplied in the response. 080 */ 081 public UnprocessableEntityException(String theMessage, Throwable theCause) { 082 super(STATUS_CODE, theMessage, theCause); 083 } 084 085 /** 086 * Constructor which accepts an array of Strings describing the issue. This strings will be translated into an {@link IBaseOperationOutcome} resource which will be supplied in the response. 087 */ 088 public UnprocessableEntityException(String... theMessage) { 089 super(STATUS_CODE, theMessage); 090 } 091}