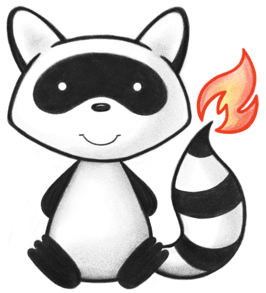
001/*- 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.system; 021 022import java.util.concurrent.TimeUnit; 023 024public final class HapiSystemProperties { 025 static final String SUPPRESS_HAPI_FHIR_VERSION_LOG = "suppress_hapi_fhir_version_log"; 026 static final String DISABLE_STATUS_BASED_REINDEX = "disable_status_based_reindex"; 027 /** 028 * This is provided for testing only! Use with caution as this property may change. 029 */ 030 static final String VALIDATION_RESOURCE_CACHE_TIMEOUT_MILLIS = "VALIDATION_RESOURCE_CACHE_EXPIRY_MS"; 031 032 static final String UNIT_TEST_CAPTURE_STACK = "unit_test_capture_stack"; 033 static final String STACKFILTER_PATTERN_PROP = "log.stackfilter.pattern"; 034 static final String HAPI_CLIENT_KEEPRESPONSES = "hapi.client.keepresponses"; 035 static final String TEST_MODE = "test"; 036 static final String UNIT_TEST_MODE = "unit_test_mode"; 037 static final long DEFAULT_VALIDATION_RESOURCE_CACHE_TIMEOUT_MILLIS = TimeUnit.MINUTES.toMillis(10); 038 static final String PREVENT_INVALIDATING_CONDITIONAL_MATCH_CRITERIA = 039 "hapi.storage.prevent_invalidating_conditional_match_criteria"; 040 static final String DISABLE_DATABASE_PARTITION_MODE_SCHEMA_CHECK = 041 "hapi.storage.disable_database_partition_mode_schema_check"; 042 043 private HapiSystemProperties() {} 044 045 /** 046 * This property is used by unit tests - do not rely on it in production code 047 * as it may change at any time. If you want to capture responses in a reliable 048 * way in your own code, just use client interceptors 049 */ 050 public static void enableHapiClientKeepResponses() { 051 System.setProperty(HAPI_CLIENT_KEEPRESPONSES, Boolean.TRUE.toString()); 052 } 053 054 public static void disableHapiClientKeepResponses() { 055 System.clearProperty(HAPI_CLIENT_KEEPRESPONSES); 056 } 057 058 /** 059 * This property is used by unit tests - do not rely on it in production code 060 * as it may change at any time. If you want to capture responses in a reliable 061 * way in your own code, just use client interceptors 062 */ 063 public static boolean isHapiClientKeepResponsesEnabled() { 064 return (Boolean.parseBoolean(System.getProperty(HAPI_CLIENT_KEEPRESPONSES))); 065 } 066 067 /** 068 * This property gets used in the logback.xml file. 069 * It causes logged stack traces to skip a number of packages that are 070 * just noise. 071 */ 072 public static void setStackFilterPattern(String thePattern) { 073 System.setProperty(STACKFILTER_PATTERN_PROP, thePattern); 074 } 075 076 /** 077 * Set the validation resource cache expireAfterWrite timeout in milliseconds 078 * 079 * @param theMillis the timeout value to set (in milliseconds) 080 */ 081 public static void setValidationResourceCacheTimeoutMillis(long theMillis) { 082 System.setProperty(VALIDATION_RESOURCE_CACHE_TIMEOUT_MILLIS, "" + theMillis); 083 } 084 085 /** 086 * Get the validation resource cache expireAfterWrite timeout in milliseconds. If it has not been set, the default 087 * value is 10 seconds. 088 */ 089 public static long getValidationResourceCacheTimeoutMillis() { 090 String property = System.getProperty(VALIDATION_RESOURCE_CACHE_TIMEOUT_MILLIS); 091 if (property == null) { 092 return DEFAULT_VALIDATION_RESOURCE_CACHE_TIMEOUT_MILLIS; 093 } 094 return Long.parseLong(property); 095 } 096 097 /** 098 * When this property is primarily used to control application shutdown behavior 099 */ 100 public static void enableTestMode() { 101 System.setProperty(TEST_MODE, Boolean.TRUE.toString()); 102 } 103 104 public static boolean isTestModeEnabled() { 105 return Boolean.parseBoolean(System.getProperty(TEST_MODE)); 106 } 107 108 /** 109 * This property is used to ensure unit test behaviour is deterministic. 110 */ 111 public static void enableUnitTestMode() { 112 System.setProperty(UNIT_TEST_MODE, Boolean.TRUE.toString()); 113 } 114 115 public static void disableUnitTestMode() { 116 System.setProperty(UNIT_TEST_MODE, Boolean.FALSE.toString()); 117 } 118 119 public static boolean isUnitTestModeEnabled() { 120 return Boolean.parseBoolean(System.getProperty(UNIT_TEST_MODE)); 121 } 122 123 /** 124 * This property prevents stack traces from getting truncated and includes the full stack trace in failed search responses. 125 */ 126 public static void enableUnitTestCaptureStack() { 127 System.setProperty(UNIT_TEST_CAPTURE_STACK, Boolean.TRUE.toString()); 128 } 129 130 public static void disableUnitTestCaptureStack() { 131 System.clearProperty(HapiSystemProperties.UNIT_TEST_CAPTURE_STACK); 132 } 133 134 public static boolean isUnitTestCaptureStackEnabled() { 135 return Boolean.parseBoolean(System.getProperty(HapiSystemProperties.UNIT_TEST_CAPTURE_STACK)); 136 } 137 138 public static boolean isDisableStatusBasedReindex() { 139 return Boolean.parseBoolean(System.getProperty(DISABLE_STATUS_BASED_REINDEX)); 140 } 141 142 public static void disableStatusBasedReindex() { 143 System.setProperty(DISABLE_STATUS_BASED_REINDEX, Boolean.TRUE.toString()); 144 } 145 146 /** 147 * This property sets the status based reindexing to disabled when the system starts up. 148 */ 149 public static void enableStatusBasedReindex() { 150 System.clearProperty(DISABLE_STATUS_BASED_REINDEX); 151 } 152 153 /** 154 * This property is used to suppress the logging of the HAPI FHIR version on startup. 155 */ 156 // TODO KHS use this in cdr 157 public static void enableSuppressHapiFhirVersionLog() { 158 System.setProperty(SUPPRESS_HAPI_FHIR_VERSION_LOG, Boolean.TRUE.toString()); 159 } 160 161 public static boolean isSuppressHapiFhirVersionLogEnabled() { 162 return Boolean.parseBoolean(System.getProperty(SUPPRESS_HAPI_FHIR_VERSION_LOG)); 163 } 164 165 public static boolean isPreventInvalidatingConditionalMatchCriteria() { 166 return Boolean.parseBoolean(System.getProperty( 167 HapiSystemProperties.PREVENT_INVALIDATING_CONDITIONAL_MATCH_CRITERIA, Boolean.FALSE.toString())); 168 } 169 170 public static boolean isDisableDatabasePartitionModeSchemaCheck() { 171 return Boolean.parseBoolean(System.getProperty( 172 HapiSystemProperties.DISABLE_DATABASE_PARTITION_MODE_SCHEMA_CHECK, Boolean.FALSE.toString())); 173 } 174}