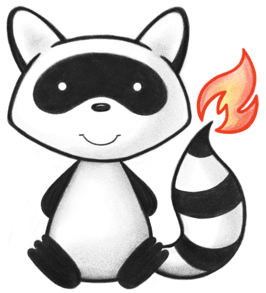
001/*- 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.tls; 021 022import ca.uhn.fhir.i18n.Msg; 023import org.apache.commons.io.FilenameUtils; 024 025import static org.apache.commons.lang3.StringUtils.isBlank; 026 027public abstract class BaseStoreInfo { 028 029 private final String myFilePath; 030 private final PathType myPathType; 031 private final char[] myStorePass; 032 private final String myAlias; 033 private final KeyStoreType myType; 034 035 public BaseStoreInfo(String theFilePath, String theStorePass, String theAlias) { 036 if (theFilePath.startsWith(PathType.RESOURCE.getPrefix())) { 037 myFilePath = theFilePath.substring(PathType.RESOURCE.getPrefix().length()); 038 myPathType = PathType.RESOURCE; 039 } else if (theFilePath.startsWith(PathType.FILE.getPrefix())) { 040 myFilePath = theFilePath.substring(PathType.FILE.getPrefix().length()); 041 myPathType = PathType.FILE; 042 } else { 043 throw new StoreInfoException(Msg.code(2117) + "Invalid path prefix"); 044 } 045 046 myStorePass = toCharArray(theStorePass); 047 myAlias = theAlias; 048 049 String extension = FilenameUtils.getExtension(theFilePath); 050 myType = KeyStoreType.fromFileExtension(extension); 051 } 052 053 public String getFilePath() { 054 return myFilePath; 055 } 056 057 public char[] getStorePass() { 058 return myStorePass; 059 } 060 061 public String getAlias() { 062 return myAlias; 063 } 064 065 public KeyStoreType getType() { 066 return myType; 067 } 068 069 public PathType getPathType() { 070 return myPathType; 071 } 072 073 protected char[] toCharArray(String theString) { 074 return isBlank(theString) ? "".toCharArray() : theString.toCharArray(); 075 } 076 077 public static class StoreInfoException extends RuntimeException { 078 private static final long serialVersionUID = 1l; 079 080 public StoreInfoException(String theMessage) { 081 super(theMessage); 082 } 083 } 084}