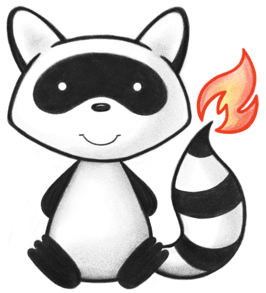
001/*- 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.util; 021 022import ca.uhn.fhir.i18n.Msg; 023import ca.uhn.fhir.rest.server.exceptions.InternalErrorException; 024import org.slf4j.Logger; 025import org.slf4j.LoggerFactory; 026 027import java.util.concurrent.CountDownLatch; 028import java.util.concurrent.TimeUnit; 029 030public class AsyncUtil { 031 private static final Logger ourLog = LoggerFactory.getLogger(AsyncUtil.class); 032 033 /** 034 * Non instantiable 035 */ 036 private AsyncUtil() {} 037 038 /** 039 * Calls Thread.sleep and if an InterruptedException occurs, logs a warning but otherwise continues 040 * 041 * @param theMillis The number of millis to sleep 042 * @return Did we sleep the whole amount 043 */ 044 public static boolean sleep(long theMillis) { 045 try { 046 Thread.sleep(theMillis); 047 return true; 048 } catch (InterruptedException theE) { 049 Thread.currentThread().interrupt(); 050 ourLog.warn("Sleep for {}ms was interrupted", theMillis); 051 return false; 052 } 053 } 054 055 public static boolean awaitLatchAndThrowInternalErrorExceptionOnInterrupt( 056 CountDownLatch theInitialCollectionLatch, long theTime, TimeUnit theTimeUnit) { 057 try { 058 return theInitialCollectionLatch.await(theTime, theTimeUnit); 059 } catch (InterruptedException e) { 060 Thread.currentThread().interrupt(); 061 throw new InternalErrorException(Msg.code(1805) + e); 062 } 063 } 064 065 public static boolean awaitLatchAndIgnoreInterrupt( 066 CountDownLatch theInitialCollectionLatch, long theTime, TimeUnit theTimeUnit) { 067 try { 068 return theInitialCollectionLatch.await(theTime, theTimeUnit); 069 } catch (InterruptedException e) { 070 Thread.currentThread().interrupt(); 071 ourLog.warn("Interrupted while waiting for latch"); 072 return false; 073 } 074 } 075}