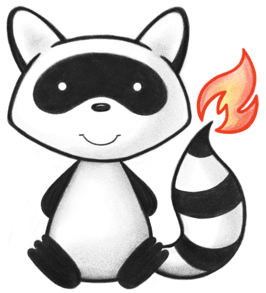
001/*- 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.util; 021 022import ca.uhn.fhir.context.BaseRuntimeChildDefinition; 023import ca.uhn.fhir.context.BaseRuntimeElementCompositeDefinition; 024import ca.uhn.fhir.context.BaseRuntimeElementDefinition; 025import ca.uhn.fhir.context.FhirContext; 026import ca.uhn.fhir.context.FhirVersionEnum; 027import ca.uhn.fhir.model.primitive.CodeDt; 028import org.apache.commons.lang3.Validate; 029import org.hl7.fhir.instance.model.api.IBase; 030import org.hl7.fhir.instance.model.api.ICompositeType; 031import org.hl7.fhir.instance.model.api.IPrimitiveType; 032 033import java.util.List; 034 035public class AttachmentUtil { 036 037 /** 038 * Fetches the base64Binary value of Attachment.data, creating it if it does not 039 * already exist. 040 */ 041 public static IPrimitiveType<byte[]> getOrCreateData(FhirContext theContext, ICompositeType theAttachment) { 042 return getOrCreateChild(theContext, theAttachment, "data", "base64Binary"); 043 } 044 045 public static IPrimitiveType<CodeDt> getOrCreateContentType(FhirContext theContext, ICompositeType theAttachment) { 046 return getOrCreateChild(theContext, theAttachment, "contentType", "code"); 047 } 048 049 public static IPrimitiveType<String> getOrCreateUrl(FhirContext theContext, ICompositeType theAttachment) { 050 return getOrCreateChild(theContext, theAttachment, "url", "uri"); 051 } 052 053 @SuppressWarnings("unchecked") 054 private static <T> IPrimitiveType<T> getOrCreateChild( 055 FhirContext theContext, ICompositeType theAttachment, String theChildName, String theChildDatatype) { 056 BaseRuntimeChildDefinition entryChild = getChild(theContext, theAttachment, theChildName); 057 List<IBase> entries = entryChild.getAccessor().getValues(theAttachment); 058 return entries.stream().map(t -> (IPrimitiveType<T>) t).findFirst().orElseGet(() -> { 059 IPrimitiveType<String> string = newPrimitive(theContext, theChildDatatype, null); 060 entryChild.getMutator().setValue(theAttachment, string); 061 return (IPrimitiveType<T>) string; 062 }); 063 } 064 065 public static void setUrl(FhirContext theContext, ICompositeType theAttachment, String theUrl) { 066 BaseRuntimeChildDefinition entryChild = getChild(theContext, theAttachment, "url"); 067 assert entryChild != null : "Version " + theContext + " has no child " + "url"; 068 String typeName = "uri"; 069 if (theContext.getVersion().getVersion().isEqualOrNewerThan(FhirVersionEnum.R4)) { 070 typeName = "url"; 071 } 072 entryChild.getMutator().setValue(theAttachment, newPrimitive(theContext, typeName, theUrl)); 073 } 074 075 public static void setContentType(FhirContext theContext, ICompositeType theAttachment, String theContentType) { 076 BaseRuntimeChildDefinition entryChild = getChild(theContext, theAttachment, "contentType"); 077 entryChild.getMutator().setValue(theAttachment, newPrimitive(theContext, "code", theContentType)); 078 } 079 080 public static void setData(FhirContext theContext, ICompositeType theAttachment, byte[] theBytes) { 081 BaseRuntimeChildDefinition entryChild = getChild(theContext, theAttachment, "data"); 082 entryChild.getMutator().setValue(theAttachment, newPrimitive(theContext, "base64Binary", theBytes)); 083 } 084 085 public static void setSize(FhirContext theContext, ICompositeType theAttachment, Integer theLength) { 086 BaseRuntimeChildDefinition entryChild = getChild(theContext, theAttachment, "size"); 087 if (theLength == null) { 088 entryChild.getMutator().setValue(theAttachment, null); 089 } else if (theContext.getVersion().getVersion().isEqualOrNewerThan(FhirVersionEnum.R5)) { 090 entryChild.getMutator().setValue(theAttachment, newPrimitive(theContext, "integer64", (long) theLength)); 091 } else { 092 entryChild.getMutator().setValue(theAttachment, newPrimitive(theContext, "unsignedInt", theLength)); 093 } 094 } 095 096 /** 097 * This is internal API- Use with caution as it may change 098 */ 099 @SuppressWarnings("unchecked") 100 static <T> IPrimitiveType<T> newPrimitive(FhirContext theContext, String theType, T theValue) { 101 BaseRuntimeElementDefinition<?> elementDefinition = theContext.getElementDefinition(theType); 102 Validate.notNull(elementDefinition, "Unknown type %s for %s", theType, theContext); 103 IPrimitiveType<T> primitive = (IPrimitiveType<T>) elementDefinition.newInstance(); 104 primitive.setValue(theValue); 105 return primitive; 106 } 107 108 /** 109 * This is internal API- Use with caution as it may change 110 */ 111 static BaseRuntimeChildDefinition getChild(FhirContext theContext, IBase theElement, String theName) { 112 BaseRuntimeElementCompositeDefinition<?> def = 113 (BaseRuntimeElementCompositeDefinition<?>) theContext.getElementDefinition(theElement.getClass()); 114 return def.getChildByName(theName); 115 } 116 117 public static ICompositeType newInstance(FhirContext theFhirCtx) { 118 return (ICompositeType) theFhirCtx.getElementDefinition("Attachment").newInstance(); 119 } 120}