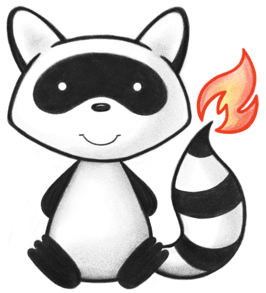
001/*- 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.util; 021 022import ca.uhn.fhir.context.FhirContext; 023import ca.uhn.fhir.context.RuntimeResourceDefinition; 024import jakarta.annotation.Nonnull; 025import org.hl7.fhir.instance.model.api.IBase; 026import org.hl7.fhir.instance.model.api.IBaseCoding; 027import org.hl7.fhir.instance.model.api.IBaseReference; 028import org.hl7.fhir.instance.model.api.IBaseResource; 029import org.hl7.fhir.instance.model.api.IIdType; 030import org.hl7.fhir.instance.model.api.IPrimitiveType; 031 032import java.util.Date; 033 034/** 035 * This class can be used to generate <code>Composition</code> resources in 036 * a version independent way. 037 * 038 * @since 6.4.0 039 */ 040public class CompositionBuilder { 041 042 private final FhirContext myCtx; 043 private final IBaseResource myComposition; 044 private final RuntimeResourceDefinition myCompositionDef; 045 private final FhirTerser myTerser; 046 047 public CompositionBuilder(@Nonnull FhirContext theFhirContext) { 048 myCtx = theFhirContext; 049 myCompositionDef = myCtx.getResourceDefinition("Composition"); 050 myTerser = myCtx.newTerser(); 051 myComposition = myCompositionDef.newInstance(); 052 } 053 054 @SuppressWarnings("unchecked") 055 public <T extends IBaseResource> T getComposition() { 056 return (T) myComposition; 057 } 058 059 /** 060 * Add a value to <code>Composition.author</code> 061 */ 062 public void addAuthor(IIdType theAuthorId) { 063 IBaseReference reference = myTerser.addElement(myComposition, "Composition.author"); 064 reference.setReference(theAuthorId.getValue()); 065 } 066 067 /** 068 * Set a value in <code>Composition.status</code> 069 */ 070 public void setStatus(String theStatusCode) { 071 myTerser.setElement(myComposition, "Composition.status", theStatusCode); 072 } 073 074 /** 075 * Set a value in <code>Composition.subject</code> 076 */ 077 public void setSubject(IIdType theSubject) { 078 myTerser.setElement(myComposition, "Composition.subject.reference", theSubject.getValue()); 079 } 080 081 /** 082 * Add a Coding to <code>Composition.type.coding</code> 083 */ 084 public void addTypeCoding(String theSystem, String theCode, String theDisplay) { 085 IBaseCoding coding = myTerser.addElement(myComposition, "Composition.type.coding"); 086 coding.setCode(theCode); 087 coding.setSystem(theSystem); 088 coding.setDisplay(theDisplay); 089 } 090 091 /** 092 * Set a value in <code>Composition.date</code> 093 */ 094 public void setDate(IPrimitiveType<Date> theDate) { 095 myTerser.setElement(myComposition, "Composition.date", theDate.getValueAsString()); 096 } 097 098 /** 099 * Set a value in <code>Composition.title</code> 100 */ 101 public void setTitle(String theTitle) { 102 myTerser.setElement(myComposition, "Composition.title", theTitle); 103 } 104 105 /** 106 * Set a value in <code>Composition.confidentiality</code> 107 */ 108 public void setConfidentiality(String theConfidentiality) { 109 myTerser.setElement(myComposition, "Composition.confidentiality", theConfidentiality); 110 } 111 112 /** 113 * Set a value in <code>Composition.id</code> 114 */ 115 public void setId(IIdType theId) { 116 myComposition.setId(theId.getValue()); 117 } 118 119 public SectionBuilder addSection() { 120 IBase section = myTerser.addElement(myComposition, "Composition.section"); 121 return new SectionBuilder(section); 122 } 123 124 public class SectionBuilder { 125 126 private final IBase mySection; 127 128 /** 129 * Constructor 130 */ 131 private SectionBuilder(IBase theSection) { 132 mySection = theSection; 133 } 134 135 /** 136 * Sets the section title 137 */ 138 public void setTitle(String theTitle) { 139 myTerser.setElement(mySection, "title", theTitle); 140 } 141 142 /** 143 * Add a coding to section.code 144 */ 145 public void addCodeCoding(String theSystem, String theCode, String theDisplay) { 146 IBaseCoding coding = myTerser.addElement(mySection, "code.coding"); 147 coding.setCode(theCode); 148 coding.setSystem(theSystem); 149 coding.setDisplay(theDisplay); 150 } 151 152 /** 153 * Adds a reference to entry.reference 154 */ 155 public void addEntry(IIdType theReference) { 156 IBaseReference entry = myTerser.addElement(mySection, "entry"); 157 entry.setReference(theReference.getValue()); 158 } 159 160 /** 161 * Adds narrative text to the section 162 */ 163 public void setText(String theStatus, String theDivHtml) { 164 IBase text = myTerser.addElement(mySection, "text"); 165 myTerser.setElement(text, "status", theStatus); 166 myTerser.setElement(text, "div", theDivHtml); 167 } 168 } 169}