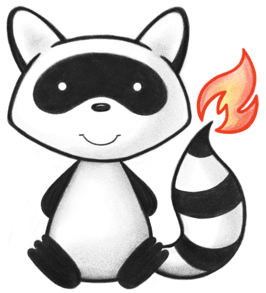
001/*- 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.util; 021 022import ca.uhn.fhir.i18n.Msg; 023import com.google.common.io.CountingInputStream; 024 025import java.io.IOException; 026import java.io.InputStream; 027 028public class CountingAndLimitingInputStream extends InputStream { 029 private final int myMaxBytes; 030 private final CountingInputStream myWrap; 031 032 @Override 033 public int read() throws IOException { 034 int retVal = myWrap.read(); 035 validateCount(); 036 return retVal; 037 } 038 039 @Override 040 public int read(byte[] b, int off, int len) throws IOException { 041 int retVal = myWrap.read(b, off, len); 042 validateCount(); 043 return retVal; 044 } 045 046 @Override 047 public int read(byte[] theRead) throws IOException { 048 int retVal = myWrap.read(theRead); 049 validateCount(); 050 return retVal; 051 } 052 053 private void validateCount() throws IOException { 054 if (myWrap.getCount() > myMaxBytes) { 055 throw new IOException(Msg.code(1807) + "Stream exceeds maximum allowable size: " + myMaxBytes); 056 } 057 } 058 059 /** 060 * Wraps another input stream, counting the number of bytes read. 061 * 062 * @param theWrap the input stream to be wrapped 063 */ 064 public CountingAndLimitingInputStream(InputStream theWrap, int theMaxBytes) { 065 myWrap = new CountingInputStream(theWrap); 066 myMaxBytes = theMaxBytes; 067 } 068}