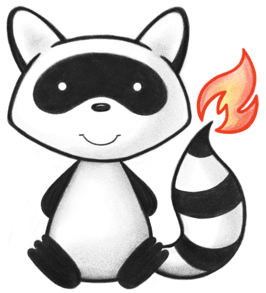
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.util; 021 022import org.hl7.fhir.instance.model.api.IPrimitiveType; 023 024import java.util.HashSet; 025import java.util.List; 026import java.util.Set; 027 028public class DatatypeUtil { 029 030 /** 031 * Convert a list of FHIR String objects to a set of native java Strings 032 */ 033 public static Set<String> toStringSet(List<? extends IPrimitiveType<?>> theStringList) { 034 HashSet<String> retVal = new HashSet<>(); 035 if (theStringList != null) { 036 for (IPrimitiveType<?> string : theStringList) { 037 if (string != null && string.getValue() != null) { 038 retVal.add(string.getValueAsString()); 039 } 040 } 041 } 042 return retVal; 043 } 044 045 /** 046 * Joins a list of strings with a single space (' ') between each string 047 */ 048 public static String joinStringsSpaceSeparated(List<? extends IPrimitiveType<String>> theStrings) { 049 StringBuilder b = new StringBuilder(); 050 for (IPrimitiveType<String> next : theStrings) { 051 if (next.isEmpty()) { 052 continue; 053 } 054 if (b.length() > 0) { 055 b.append(' '); 056 } 057 b.append(next.getValue()); 058 } 059 return b.toString(); 060 } 061 062 /** 063 * Returns {@link IPrimitiveType#getValueAsString()} if <code>thePrimitiveType</code> is 064 * not null, else returns null. 065 */ 066 public static String toStringValue(IPrimitiveType<?> thePrimitiveType) { 067 return thePrimitiveType != null ? thePrimitiveType.getValueAsString() : null; 068 } 069 070 /** 071 * Returns {@link IPrimitiveType#getValue()} if <code>thePrimitiveType</code> is 072 * not null, else returns null. 073 */ 074 public static Boolean toBooleanValue(IPrimitiveType<Boolean> thePrimitiveType) { 075 return thePrimitiveType != null ? thePrimitiveType.getValue() : null; 076 } 077}