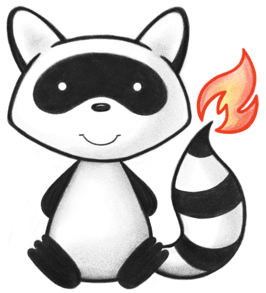
001/*- 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.util; 021 022import ca.uhn.fhir.rest.param.DateRangeParam; 023import jakarta.annotation.Nonnull; 024import jakarta.annotation.Nullable; 025 026import java.util.Date; 027 028public class DateRangeUtil { 029 030 /** 031 * Narrow the DateRange to be within theStartInclusive, and theEndExclusive, if provided. 032 * 033 * @param theDateRangeParam the initial range, null for unconstrained 034 * @param theStartInclusive a lower bound to apply, or null for unchanged. 035 * @param theEndExclusive an upper bound to apply, or null for unchanged. 036 * @return a DateRange within the original range, and between theStartInclusive and theEnd 037 */ 038 @Nonnull 039 public static DateRangeParam narrowDateRange( 040 @Nullable DateRangeParam theDateRangeParam, 041 @Nullable Date theStartInclusive, 042 @Nullable Date theEndExclusive) { 043 if (theStartInclusive == null && theEndExclusive == null) { 044 return theDateRangeParam; 045 } 046 DateRangeParam result = 047 theDateRangeParam == null ? new DateRangeParam() : new DateRangeParam(theDateRangeParam); 048 049 Date startInclusive = theStartInclusive; 050 if (startInclusive != null) { 051 Date inputStart = result.getLowerBoundAsInstant(); 052 053 Date upperBound = result.getUpperBoundAsInstant(); 054 if (upperBound != null && upperBound.before(startInclusive)) { 055 startInclusive = upperBound; 056 } 057 058 if (theDateRangeParam == null || inputStart == null || inputStart.before(startInclusive)) { 059 result.setLowerBoundInclusive(startInclusive); 060 } 061 } 062 if (theEndExclusive != null) { 063 Date inputEnd = result.getUpperBound() == null 064 ? null 065 : result.getUpperBound().getValue(); 066 if (theDateRangeParam == null || inputEnd == null || inputEnd.after(theEndExclusive)) { 067 result.setUpperBoundExclusive(theEndExclusive); 068 } 069 } 070 071 return result; 072 } 073}