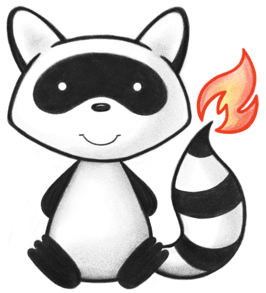
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.util; 021 022import ca.uhn.fhir.i18n.Msg; 023import ca.uhn.fhir.model.api.ICompositeElement; 024import ca.uhn.fhir.model.api.IElement; 025import org.hl7.fhir.instance.model.api.IBase; 026 027import java.util.ArrayList; 028import java.util.List; 029 030public class ElementUtil { 031 032 @SuppressWarnings("unchecked") 033 public static boolean isEmpty(Object... theElements) { 034 if (theElements == null) { 035 return true; 036 } 037 for (int i = 0; i < theElements.length; i++) { 038 Object next = theElements[i]; 039 if (next instanceof List) { 040 if (!isEmpty((List<? extends IBase>) next)) { 041 return false; 042 } 043 } else if (next instanceof String && (!((String) next).isEmpty())) { 044 return false; 045 } else if (next != null && !((IBase) next).isEmpty()) { 046 return false; 047 } 048 } 049 return true; 050 } 051 052 public static boolean isEmpty(IBase... theElements) { 053 if (theElements == null) { 054 return true; 055 } 056 for (int i = 0; i < theElements.length; i++) { 057 IBase next = theElements[i]; 058 if (next != null && !next.isEmpty()) { 059 return false; 060 } 061 } 062 return true; 063 } 064 065 public static boolean isEmpty(IElement... theElements) { 066 if (theElements == null) { 067 return true; 068 } 069 for (int i = 0; i < theElements.length; i++) { 070 IBase next = theElements[i]; 071 if (next != null && !next.isEmpty()) { 072 return false; 073 } 074 } 075 return true; 076 } 077 078 public static boolean isEmpty(List<? extends IBase> theElements) { 079 if (theElements == null) { 080 return true; 081 } 082 for (int i = 0; i < theElements.size(); i++) { 083 IBase next; 084 try { 085 next = theElements.get(i); 086 } catch (ClassCastException e) { 087 List<?> elements = theElements; 088 String s = "Found instance of " + elements.get(i).getClass() 089 + " - Did you set a field value to the incorrect type? Expected " + IBase.class.getName(); 090 throw new ClassCastException(Msg.code(1748) + s); 091 } 092 if (next != null && !next.isEmpty()) { 093 return false; 094 } 095 } 096 return true; 097 } 098 099 /** 100 * Note that this method does not work on HL7.org structures 101 */ 102 public static <T extends IElement> List<T> allPopulatedChildElements(Class<T> theType, Object... theElements) { 103 ArrayList<T> retVal = new ArrayList<T>(); 104 for (Object next : theElements) { 105 if (next == null) { 106 continue; 107 } else if (next instanceof IElement) { 108 addElement(retVal, (IElement) next, theType); 109 } else if (next instanceof List) { 110 for (Object nextElement : ((List<?>) next)) { 111 if (!(nextElement instanceof IBase)) { 112 throw new IllegalArgumentException( 113 Msg.code(1749) + "Found element of " + nextElement.getClass()); 114 } 115 addElement(retVal, (IElement) nextElement, theType); 116 } 117 } else { 118 throw new IllegalArgumentException(Msg.code(1750) + "Found element of " + next.getClass()); 119 } 120 } 121 return retVal; 122 } 123 124 // @SuppressWarnings("unchecked") 125 private static <T extends IElement> void addElement(ArrayList<T> retVal, IElement next, Class<T> theType) { 126 if (theType != null && theType.isAssignableFrom(next.getClass())) { 127 retVal.add(theType.cast(next)); 128 } 129 if (next instanceof ICompositeElement) { 130 ICompositeElement iCompositeElement = (ICompositeElement) next; 131 // TODO: Use of a deprecated method should be resolved. 132 retVal.addAll(iCompositeElement.getAllPopulatedChildElementsOfType(theType)); 133 } 134 } 135}