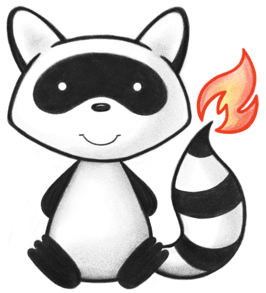
001/* 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2025 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.util; 021 022import org.apache.commons.lang3.builder.CompareToBuilder; 023import org.apache.commons.lang3.builder.EqualsBuilder; 024import org.apache.commons.lang3.builder.HashCodeBuilder; 025 026public class FhirVersionIndependentConcept implements Comparable<FhirVersionIndependentConcept> { 027 028 private final String mySystem; 029 private final String mySystemVersion; 030 private final String myCode; 031 private final String myDisplay; 032 private int myHashCode; 033 034 /** 035 * Constructor 036 */ 037 public FhirVersionIndependentConcept(String theSystem, String theCode) { 038 this(theSystem, theCode, null); 039 } 040 041 public FhirVersionIndependentConcept(String theSystem, String theCode, String theDisplay) { 042 this(theSystem, theCode, theDisplay, null); 043 } 044 045 public FhirVersionIndependentConcept(String theSystem, String theCode, String theDisplay, String theSystemVersion) { 046 mySystem = theSystem; 047 mySystemVersion = theSystemVersion; 048 myCode = theCode; 049 myDisplay = theDisplay; 050 myHashCode = new HashCodeBuilder(17, 37).append(mySystem).append(myCode).toHashCode(); 051 } 052 053 public String getDisplay() { 054 return myDisplay; 055 } 056 057 public String getSystem() { 058 return mySystem; 059 } 060 061 public String getSystemVersion() { 062 return mySystemVersion; 063 } 064 065 public String getCode() { 066 return myCode; 067 } 068 069 @Override 070 public boolean equals(Object theO) { 071 if (this == theO) { 072 return true; 073 } 074 075 if (theO == null || getClass() != theO.getClass()) { 076 return false; 077 } 078 079 FhirVersionIndependentConcept that = (FhirVersionIndependentConcept) theO; 080 081 return new EqualsBuilder() 082 .append(mySystem, that.mySystem) 083 .append(myCode, that.myCode) 084 .isEquals(); 085 } 086 087 @Override 088 public int hashCode() { 089 return myHashCode; 090 } 091 092 @Override 093 public int compareTo(FhirVersionIndependentConcept theOther) { 094 CompareToBuilder b = new CompareToBuilder(); 095 b.append(mySystem, theOther.getSystem()); 096 b.append(myCode, theOther.getCode()); 097 return b.toComparison(); 098 } 099 100 @Override 101 public String toString() { 102 return "[" + mySystem + "|" + myCode + "]"; 103 } 104}