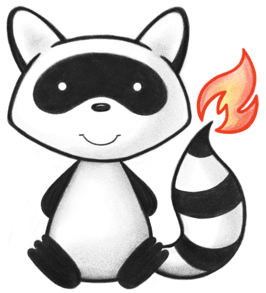
001/*- 002 * #%L 003 * HAPI FHIR - Core Library 004 * %% 005 * Copyright (C) 2014 - 2024 Smile CDR, Inc. 006 * %% 007 * Licensed under the Apache License, Version 2.0 (the "License"); 008 * you may not use this file except in compliance with the License. 009 * You may obtain a copy of the License at 010 * 011 * http://www.apache.org/licenses/LICENSE-2.0 012 * 013 * Unless required by applicable law or agreed to in writing, software 014 * distributed under the License is distributed on an "AS IS" BASIS, 015 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 016 * See the License for the specific language governing permissions and 017 * limitations under the License. 018 * #L% 019 */ 020package ca.uhn.fhir.util; 021 022import com.google.common.collect.ArrayListMultimap; 023import com.google.common.collect.ImmutableSet; 024import com.google.common.collect.ListMultimap; 025 026import java.util.Set; 027import java.util.function.BiConsumer; 028import java.util.function.BinaryOperator; 029import java.util.function.Function; 030import java.util.function.Supplier; 031import java.util.stream.Collector; 032 033/** 034 * Copied from https://stackoverflow.com/questions/23003542/cleanest-way-to-create-a-guava-multimap-from-a-java-8-stream 035 */ 036public class MultimapCollector<T, K, V> implements Collector<T, ListMultimap<K, V>, ListMultimap<K, V>> { 037 038 private final Function<T, K> keyGetter; 039 private final Function<T, V> valueGetter; 040 041 public MultimapCollector(Function<T, K> keyGetter, Function<T, V> valueGetter) { 042 this.keyGetter = keyGetter; 043 this.valueGetter = valueGetter; 044 } 045 046 public static <T, K, V> MultimapCollector<T, K, V> toMultimap( 047 Function<T, K> keyGetter, Function<T, V> valueGetter) { 048 return new MultimapCollector<>(keyGetter, valueGetter); 049 } 050 051 public static <T, K, V> MultimapCollector<T, K, T> toMultimap(Function<T, K> keyGetter) { 052 return new MultimapCollector<>(keyGetter, v -> v); 053 } 054 055 @Override 056 public Supplier<ListMultimap<K, V>> supplier() { 057 return ArrayListMultimap::create; 058 } 059 060 @Override 061 public BiConsumer<ListMultimap<K, V>, T> accumulator() { 062 return (map, element) -> map.put(keyGetter.apply(element), valueGetter.apply(element)); 063 } 064 065 @Override 066 public BinaryOperator<ListMultimap<K, V>> combiner() { 067 return (map1, map2) -> { 068 map1.putAll(map2); 069 return map1; 070 }; 071 } 072 073 @Override 074 public Function<ListMultimap<K, V>, ListMultimap<K, V>> finisher() { 075 return map -> map; 076 } 077 078 @Override 079 public Set<Characteristics> characteristics() { 080 return ImmutableSet.of(Characteristics.IDENTITY_FINISH); 081 } 082}